Coding efficiency for beginners: write reusable code
Embrace the secrets of being lazy. Learn how to write less code while getting more done. Become adored by your project manager for finishing projects faster. Lazy coder Aaron Morris, interactive developer at Jam3, gets you started
I’m going to let you in on a secret. You have to promise you won’t share it with anyone. OK? Here it isb... The best coders write the least amount of code. Sounds a little counter intuitive doesn’t it? But it's not. Now an experienced coder might be writing 3D engines which are obviously many more lines of code than someone writing a menu. What I'm getting at is that if both a junior and senior developer solve the same problem, the senior developer would have written less code. The reason is that the senior developer has learned how to be lazy. They’ve learned how to code efficiently and how to leverage old code to be reusable. In this article I'm going to share with you some of the tips and tricks I've picked up over the years.
Not every line of code is unique
There is a common mantra in the coding world. Write reusable code. You see it on job ads, tutorials, coding articles (like this one), and so on … There is good reason for this. The more you can reuse the less you’ll have to write every time. This saves time and money which is always a good thing. However, for all this talk about making sure code is reusable it can actually be a difficult concept for new coders to wrap their heads around. Inexperienced coders typically go one of two ways on this. Either they look at their code and think everything they’ve done is a one off situation and none of it will be needed again or they go overboard and try to make everything reusable. It's this first scenario that we’ll be tackling first.
It's very easy to look at a large block of code and say to yourself “oh, this code is unique, I’ll never need to use this again” and you’d probably be right. However, the trick to being lazy is to break down the code into smaller chunks and look again. Look for the little things that seem simple but are things that need to be done all the time. Maybe they only take a line or two of code but these small things add up over time.
One thing that is always an issue in Flash is making sure that text is on whole pixels. If your textField is not on a whole pixel then the text won’t be rendered correctly.
myTextField.x = 12; //instead ofmyTextField.x = 12.2;
To fix this problem you could write this:
myTextField.x = int(12.7);
Pretty simple except that you’d have cast the value as an integer every time you wanted to place text.
The reusable way is to create a base class for your TextFields and to use that every time you need some text.
Get the Creative Bloq Newsletter
Daily design news, reviews, how-tos and more, as picked by the editors.
public class WholePixelText extends TextField { public function WholePixelText () { super(); } public override function set x(value:Number):void { super.x = int(value); } public override function set y(value:Number):void { super.y = int(value); } }
Now instead of going new TextField(); you would say new WholePixelText(); and then never worry again that your text could be on a half pixel. No matter what we set the x and y properties to, they will always be floored to whole numbers. We have just saved ourselves countless seconds and keystrokes from typing int(xVal) over and over again. Not only that, we’ve eliminated a failure point by ensuring that our text will be done properly.
Another way to reduce the amount of code you write is to save important functions. Math functions are something that I always have difficulty remembering so I’ve gotten into the habit of saving important methods for late. For example, how many times have you needed a random number between two integers?
You could try to remember this:
var myRand:int =Math.random()*(max-min)+min;
or you could write this once and then add a static Utils class that contains this:
public static function randomInt(min:int=0,max:int=1):int { return Math.random()*(max-min)+min; } //Then the next time you need a random int //this is all you need to remember:var myRand:int = Utils.randomInt(min,max);
You could also include functions for getting the angle between two points or converting degrees to radians and back again. These are important functions that you’ll find yourself using over and over again. No need to write them every time from scratch. Embrace the lazy, write once. Use everywhere.
It's also important to be able to identify where things look very different graphically but in reality share a lot of the same underlying code. A great example of this is buttons. Buttons can look very different but right down deep they all have some common elements. They all have hit areas and rollover effects. A great way to handle this is to write a common base class for all the buttons you use. This way you can eliminate some of the repetitive tasks associated with creating buttons.
Here’s an example of a basic button base class:
public class ButtonBase extends Sprite { public function ButtonBase() { setupHit(); this.buttonMode=true; this.mouseEnabled=true; this.mouseChildren=false; this.addEventListener(MouseEvent.ROLL_OVER, onOver, false, 0, true); this.addEventListener(MouseEvent.ROLL_OUT, onOut, false, 0, true); } protected function setupHit():void { this.graphics.clear(); this.graphics.beginFill(0xffffff, 0); this.graphics.drawRect(0, 0, this.width, this.height); this.graphics.endFill(); } protected function onOver(e:Event):void { } protected function onOut(e:Event):void { } }
Then to customise it for your particular button you would just override the onOver and onOut functions to create your own effects. This saves a lot of time since you don’t have to worry about the hit area, making sure buttonMode is enabled or adding listeners for roll overs.
Don't make everything reusable
The other mistake, that I mentioned before, that a lot of coders make is to try and make everything they do reusable. In theory this is a great idea but in reality you can end up doing more work than if you just wrote it from scratch when you needed it which goes against our lazy ideals.
A great example of this happening is the code scroll bar. Scroll bars come in all kinds of shapes and sizes. It makes sense to try and write code for a scroll bar that you can reuse again and again. The scroll bar trap comes from trying to make a scroll bar that does too much or is too hard to use. Most scroll bars are either vertical or horizontal. My wild guesstimate is that 98 per cent of all scroll bars are used in one of these two configurations with a heavy leaning towards vertical. However, when writing code like this the temptation for some is to make a scroll bar that can be used on any angle, be it 90, 180 or 127. 90 and 180 are no brainers but it doesn’t really make sense to spend hours/days coding something that will be used so rarely.
The lazy coder we are striving to be would never do this. The lazy coder doesn’t worry about crazy edge cases. They code for the vast majority of cases and worry about the one offs when they come.
Hopefully with these tips and ideas you can start thinking the lazy way. Save those key strokes and stop wasting valuable time by writing extra code. Keep all these things in mind and you too can be The Lazy Coder.
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
The Creative Bloq team is made up of a group of design fans, and has changed and evolved since Creative Bloq began back in 2012. The current website team consists of eight full-time members of staff: Editor Georgia Coggan, Deputy Editor Rosie Hilder, Ecommerce Editor Beren Neale, Senior News Editor Daniel Piper, Editor, Digital Art and 3D Ian Dean, Tech Reviews Editor Erlingur Einarsson and Ecommerce Writer Beth Nicholls and Staff Writer Natalie Fear, as well as a roster of freelancers from around the world. The 3D World and ImagineFX magazine teams also pitch in, ensuring that content from 3D World and ImagineFX is represented on Creative Bloq.
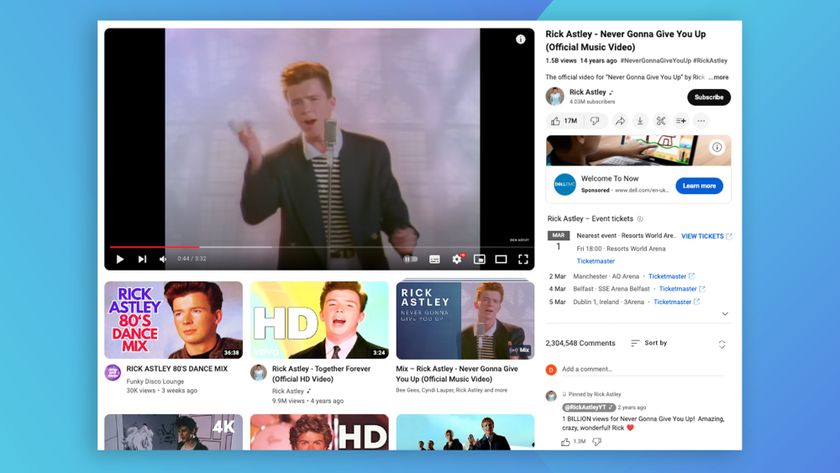
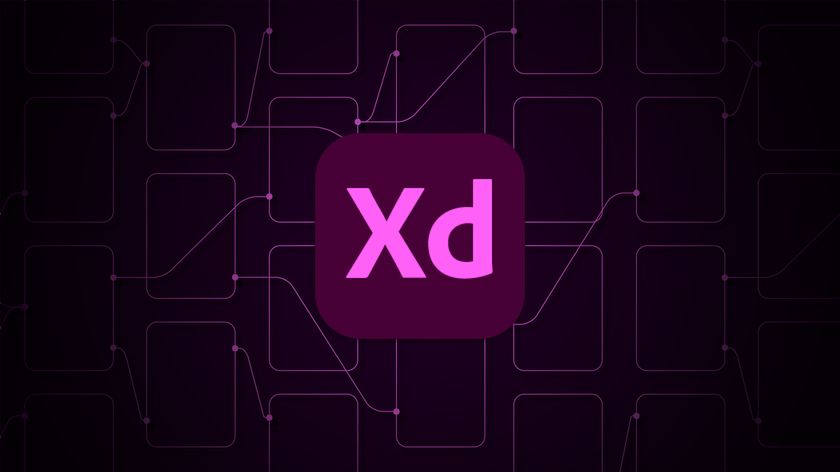
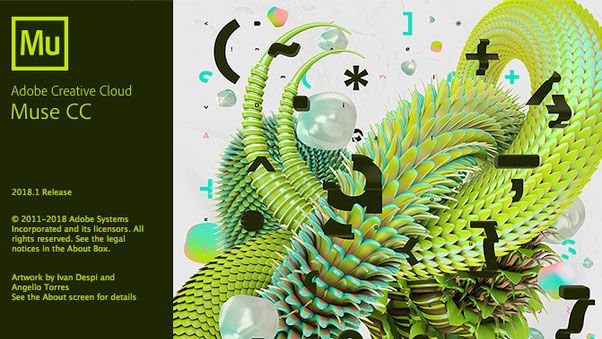
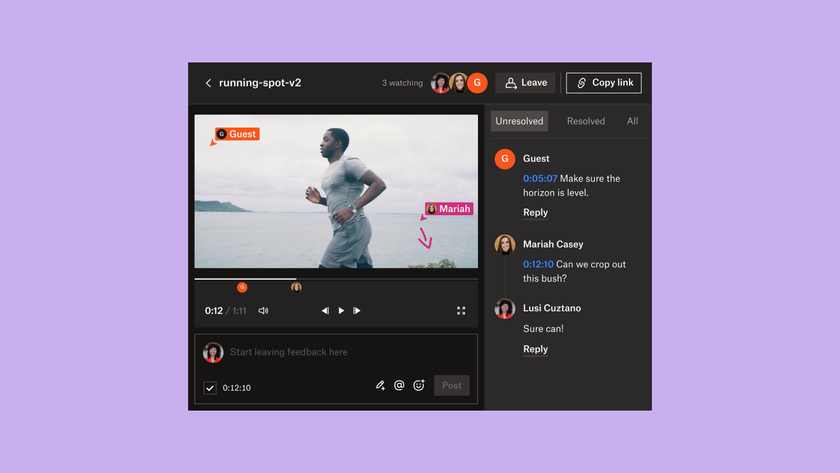
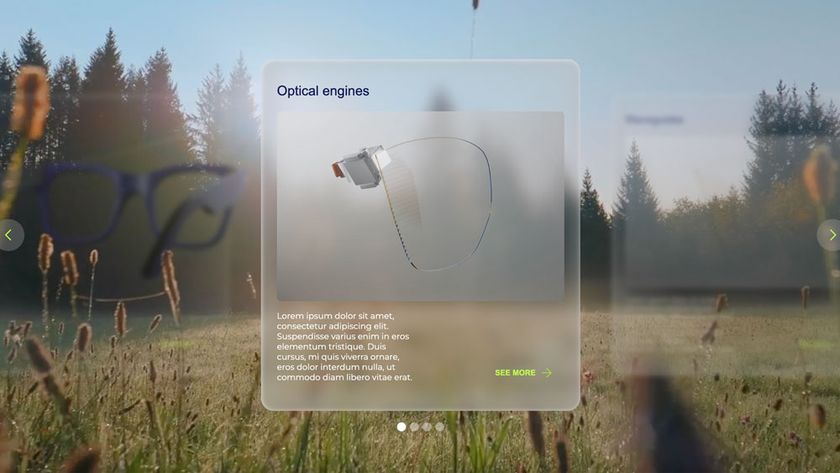
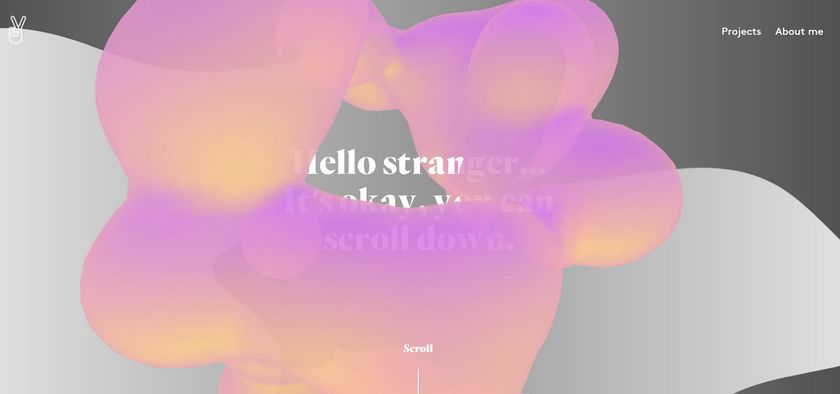
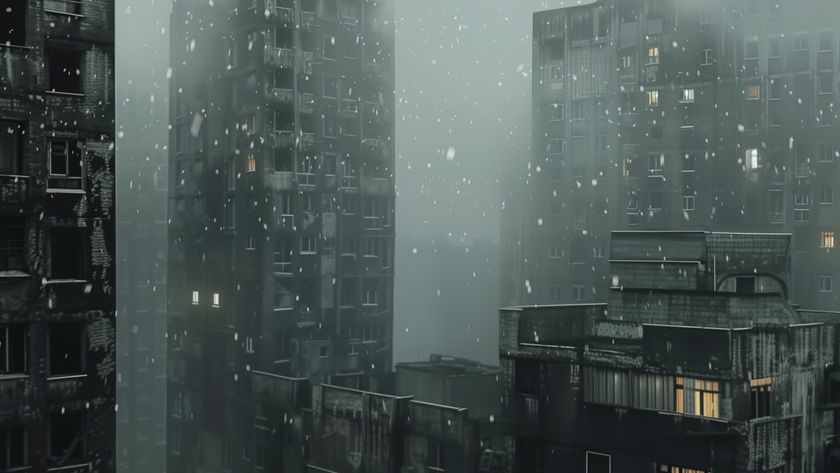
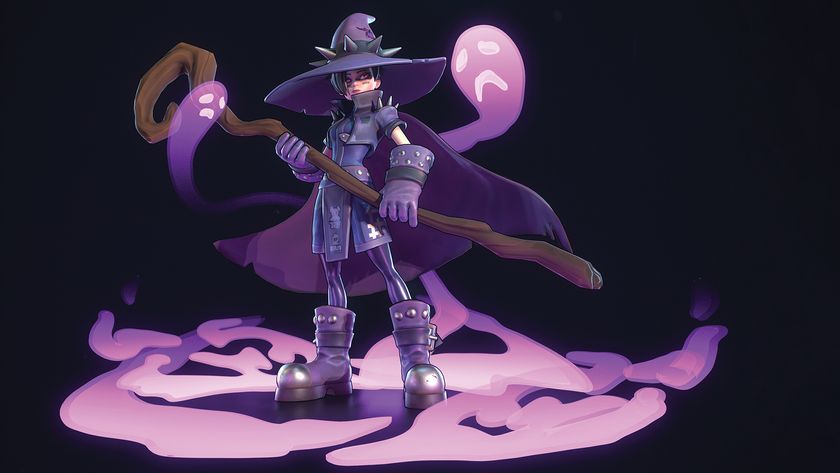
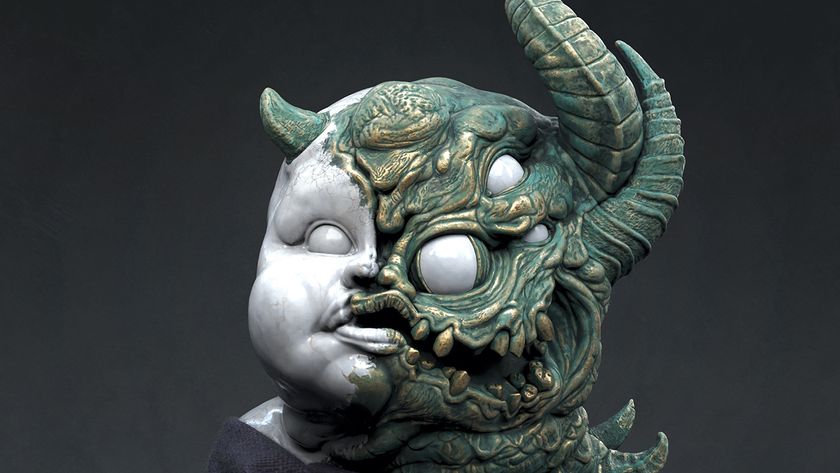
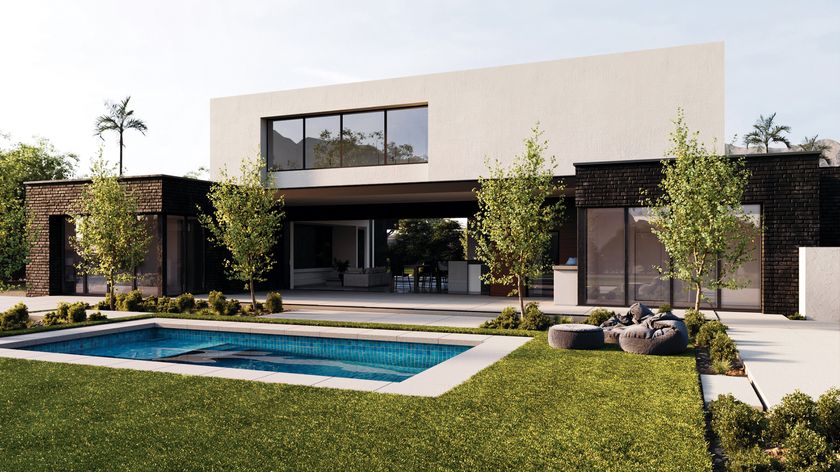
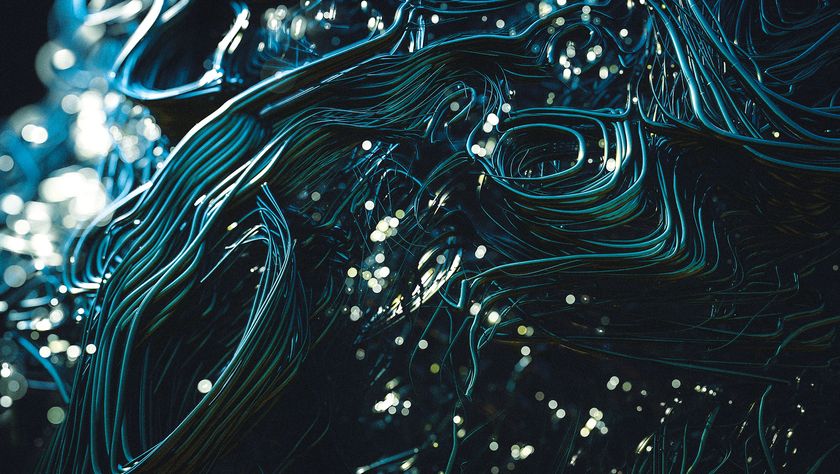
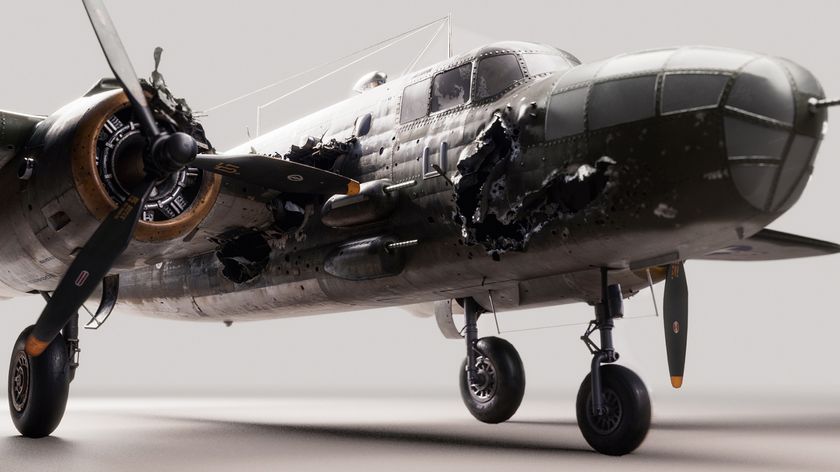