Link users to geolocation data with HTML5
Christopher Schmitt, author of CSS Cookbook and co-founder of Environments for Humans, explains how to use the HTML5 GeoLocation jQuery Plugin
Using geolocation, site builders can now enable visitors to interact with content and applications in new ways. In this tutorial, I’ll walk you through how to use the new HTML5 GeoLocation jQuery Plugin – which works even when geolocation isn’t supported by the user’s browser – along with address information written in hCard microformats, to indicate which locations are closest.
Our project aims to enable attendees at the annual SXSW Interactive Festival in Austin, Texas to track down a good restaurant. We’ll use the HTML5 GeoLocation jQuery Plugin that I created to pull in addresses of the best BBQ restaurants in the state capital, using data compiled by the kind folks at theqcard.com. (Obviously, you won’t be able to use this on the other side of the Atlantic, so I’ve also put together a UK demo so you can see how it works in practice. Okay, let’s get started. Firstly, using the easy-to-remember HTML5 Doctype, create a basic HTML5 web page:
<!DOCTYPE html> <head> <meta charset="UTF-8" /> <title>Austin BBQ Locations</title> </head> <body> </body> </html>
While IE9 supports HTML5 natively, older versions don’t respect the new HTML elements. To get round this, we’ll use JavaScript with conditional comments to force IE to create new elements based on HTML5’s element names.
JavaScript
Thanks to hosts like Google providing a CDN (Content Delivery Network), we can easily associate this JavaScript library in between the <head> elements without supporting additional files ourselves:
<!--[if lt IE 9]> <script src="http://html5shim.googlecode.com/svn/trunk/html5.js"></script> <![endif]-->
(By the way, if you’re using Modernizr the HTML5 shim is already baked into it.)
Despite jQuery’s immense popularity, humans are prone to error. Be honest: how many times have you been troubleshooting only to realise you made the simplest mistake of forgetting to associate jQuery to a web page? For this reason, the HTML5 GeoLocation jQuery Plugin checks to make sure you have the jQuery library installed! And, again thanks to Google CDN, we can easily add the jQuery library without hosting the asset ourselves, using the following code:
<!--[if lt IE 9]> <script src="http://html5shim.googlecode.com/svn/trunk/html5.js"></script> <![endif]--> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
Microformats
Microformats are design patterns for common pieces of information that simple HTML elements can’t handle on their own. From CVs to calendar events, there are several standard microformats for almost any type of situation. For this project, we’ll use the hCard microformat, which is based on the vCard specification, for the restaurant locations:
Daily design news, reviews, how-tos and more, as picked by the editors.
<div class="vcard"> <a class="url fn n" href="http://www.facebook.com/profile.php?id=100000471620703"> <span class="given-name">Jane</span> <span class="additional-name"></span> <span class="family-name">Smith</span> </a> <div class="org">Old School BBQ and Grill</div> <div class="adr"> <div class="street-address">2907 E MLK Jr Blvd.</div> <span class="locality">Austin</span>, <span class="region">TX</span>, <span class="postal-code">78702</span> <span class="country-name">USA</span> </div> <div class="tel">512-974-6830</div> </div>
If needed, use the hCard Generator to help create the markup for each of the business locations.
Next, we’ll insert a placeholder for distance in each address. The HTML5 GeoLocation script sorts through the list of addresses, but also displays an estimate of how far away each location is to the user.
<div class="vcard"> <a class="url fn n" href="http://www.theqcard.com/"> <span class="given-name">Jane</span> <span class="additional-name"></span> <span class="family-name">Smith</span> </a> <div class="org">Old School BBQ and Grill</div> <div class="adr"> <div class="street-address">2907 E MLK Jr Blvd.</div> <span class="locality">Austin</span>, <span class="region">TX</span>, <span class="postal-code">78702</span> <span class="country-name">USA</span> </div> <div class="tel">512-974-6830</div> <div class="note distance"> </div> </div>
To apply structure to a list of addresses, put the addresses in an unordered list using the ol and li elements. Then wrap the list with a div with an id attribute set to locations2 (the accepted default value is locations):
<div id="locations2"> <ol> <li><!-- Microformats address 1 --></li> <li><!-- Microformats address 2 --></li> <li><!-- Microformats address 3 --></li> <li><!-- Microformats address 4 --></li> ... </ol></div>
Feedback
While the HTML5 GeoLocation jQuery Plugin is processing the addresses and figuring out which is closest, there is going to be a delay. So, to give some feedback to the user place a div element with a class attribute of notification.
Feel free to style this notification text any way you like through CSS – or even put in an Ajax-style loading spinner graphic:
<div class="notification" style="display:none">please wait... sorting addresses based on your location</div>
Next, we want to apply an empty h2 element with an id attribute of geodata that holds the address location of the site visitor. Again, feel free to use CSS to style the text for the location to match your site’s style:
<h2 id="geodata"></h2>
The latest version of the jQuery Plugin can be found at GitHub. Download and associate the jQuery Plugin to your web page:
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script><script type="text/javascript" src="js/jquery-location.1.0.0.js"></script>
Google Maps API
Next, sign up to the Google Maps API at code.google.com/apis/maps/signup.html and register the appropriate domain.
For example, if you intend to use the GeoLocation jQuery Plugin on example.com, enter http://example.com as My Web Site URL. (Oh, and be sure to read those terms and conditions!). The API key is about 87 characters at the time of this writing.
With everything installed, we want to tell the HTML5 GeoLocation jQuery Plugin where to look for the list of addresses in the web page.
Since we wrapped a div with an id attribute value of locations2, it’s a simple matter of calling the plug-in and telling it where to look within the web page:
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script><script type="text/javascript" src="js/jquery-location.1.0.0.js"></script> <script type="text/javascript"> $(document).ready(function() { $('#locations2').location({ }); });</script>
If the Google API Key isn’t already provided (for example, for use of other Google online services), you need to set the plug-in apiKey option:
<script type="text/javascript"> $(document).ready(function() { $('#locations2').location({ 'apiKey' : 'YOUR_API_KEY_HERE' }); });</script>
For speedier performance, you can reduce the number of trips to Google to geoencode the postal addresses. The longitude and latitude for addresses can be found out through free online services such as worldKit geocoder or Geocoder.us.
Using the HTML5 data attribute, list out information within the hCard microformat’s div element with an adr attribute value:
<div class="adr" data-longitude="-97.723410" data-latitude="30.262098"
data-lang="js">
The HTML5 GeoLocation jQuery Plugin has been set up to be flexible so that it can work with simple and customisable id and class selectors. Feel free to adjust the id and class attributes the plug-in looks for within your pages' markup.
$('#locations').location( { apiKey: 'YOUR_API_KEY_HERE', geodata: '#geodata', notification: '.notification', recheck: '.recheck', distance: '.distance', geoAdr: '.geoAdr', listElement: 'li' } );
With the HTML5 GeoLocation jQuery Plugin set up, you can now cater the design of geolocation elements with simple id and class selectors.
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
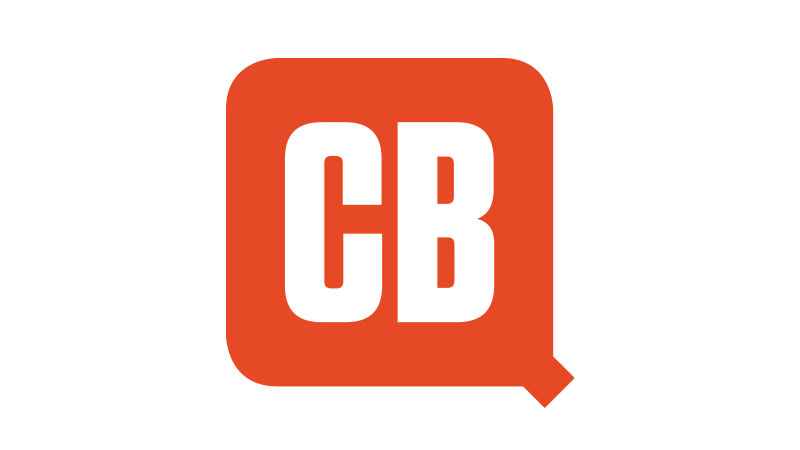
The Creative Bloq team is made up of a group of art and design enthusiasts, and has changed and evolved since Creative Bloq began back in 2012. The current website team consists of eight full-time members of staff: Editor Georgia Coggan, Deputy Editor Rosie Hilder, Ecommerce Editor Beren Neale, Senior News Editor Daniel Piper, Editor, Digital Art and 3D Ian Dean, Tech Reviews Editor Erlingur Einarsson, Ecommerce Writer Beth Nicholls and Staff Writer Natalie Fear, as well as a roster of freelancers from around the world. The ImagineFX magazine team also pitch in, ensuring that content from leading digital art publication ImagineFX is represented on Creative Bloq.