What’s new in Angular 4?
Learn how to build an app using the exciting new features in the JavaScript framework.
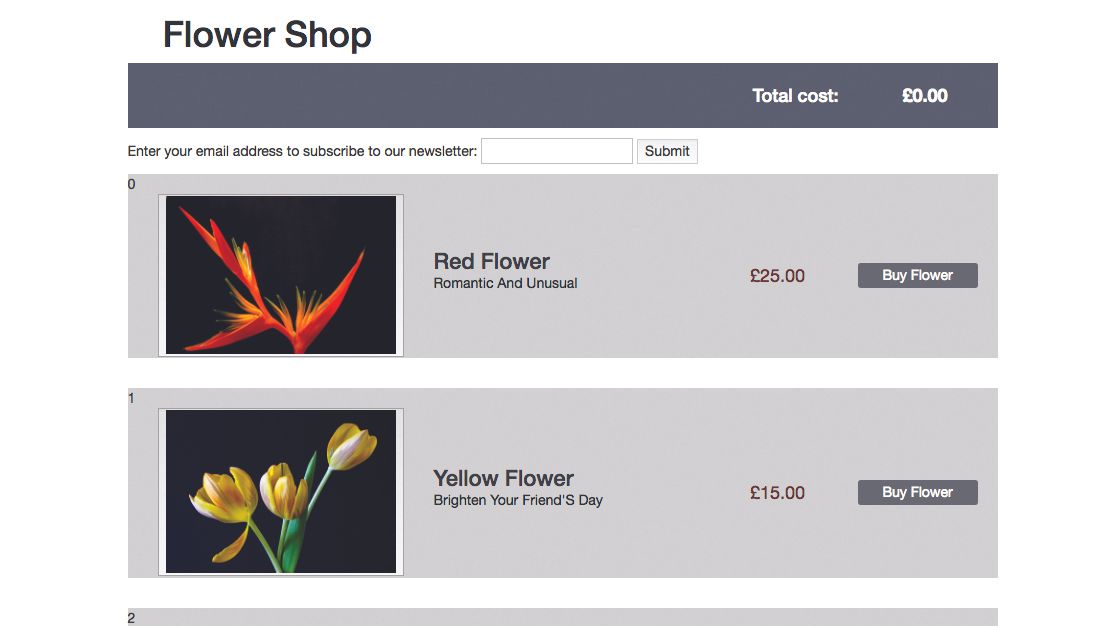
In March, the Angular team released version 4, and with it some exciting new features to explore. In this tutorial we’ll show you how to make an app using some of these new features, as well as demonstrating some of the powerful performance-enhancing, under- the-hood changes.
What's new?
The Angular team is calling it an ‘invisible makeover’, because most of the changes are in the background rather than with core coding functionality. An important change is the move to ahead-of-time compilation as standard, which has the potential to drastically improve performance when used correctly. TypeScript 2.1+ is also now supported, which gives us access to all the new features of ES2015.
That’s not to say there aren’t also any notable changes to the basics – for example, there's a useful new template-binding syntax that enables us to simplify our code by adding an else option to ngIf, and the ability to assign local variables within an ngFor.
Did I miss 3.0?
Angular 4 is the first major version release of the framework adopted of semantic versioning. So don’t worry, you didn’t miss 3.0 – there were two major updates, and both were rolled into version 4.0.
Note also that Angular 1.x is now known as AngularJS, and Angular 2+ is simply referred to as Angular. Be wary as some third-party tutorials and libraries may not have updated their use of these terms.
Get the Creative Bloq Newsletter
Daily design news, reviews, how-tos and more, as picked by the editors.
Get the tutorial files
In this tutorial, we'll be working with a Flower Shop app. To download the example app, go to FileSilo, select Free Stuff and Free Content next to the tutorial. Note: First time users will have to register to use FileSilo. Once you're logged in, you can download the example app here.
Let's get started!
01. Start with Node
Let's starting from scratch, and use the Angular CLI to build a Hello World app. If you want to update an existing app, skip to step 4. If you haven’t already, download Node, which comes pre-packaged with npm. If you already have Node, check it's at least Node 6.9.x and npm 3.x.x from the command line.
$ node -v
v6.10.2
$ npm -v
3.10.10
02. Set up a new project
Now we have a package manager we can use it to install Angular and the Angular CLI. Amongst other things, the CLI enables you to easily generate new projects and components.
$ npm install -g @angular/cli
$ ng new my-first-angular4-app # Angular CLI commands use 'ng'
$ ng serve -open # This command will bootstrap your app and launch it in the browser
03. Check versioning
If you’ve not seen an Angular project before, take time to familiarise yourself with the file structure generated by the CLI. For our new project, our package.json should list version 4.0.0 Angular packages.
"dependencies": {
"@angular/common": "^4.0.0",
"@angular/compiler": "^4.0.0",
"@angular/core": "^4.0.0",
"@angular/forms": "^4.0.0",
"@angular/http": "^4.0.0",
"@angular/platform-browser": "^4.0.0",
"@angular/platform-browser-dynamic": "^4.0.0",
"@angular/router": "^4.0.0" [...]}
04. Upgrade to Angular 4
If you have an existing Angular app with 2.x versions listed, it’s really easy to update to version 4 in most cases. We just need to install and update the relevant packages from the command line. Mac:
$ npm install @angular/{common,compiler,compiler-cli,core,forms,http,platform-browser,platform-browser-dynamic,platform-server,router,animations}@latest typescript@latest --save
Windows:
> npm install @angular/common@latest @angular/compiler@latest @angular/compiler-cli@latest @angular/core@latest @angular/forms@latest @angular/http@latest @angular/platform-browser@latest @angular/platform-browser-dynamic@latest @angular/platform-server@latest @angular/router@latest @angular/animations@latest typescript@latest --save
05. Download the tutorial app
From now on we’ll be working with an Angular 2 example app that you can download from FileSilo (for full notes on how to do this, see intro above). Note that this app has been created purely for illustrative purposes. Once you’ve downloaded theFlower Shop app into your root directory, install dependencies and launch in the browser.
$ npm install
$ ng serve --open
06. Upgrade Flower Shop to 4.0
Within the flower-shop directory, upgrade to 4.0. We have some UNMET PEER DEPENDENCY errors after upgrading.
07. Fix peer dependencies
Peer dependencies are for managing projects that have packages relying on different versions of the same dependencies. You have to add these manually to your package.json file. The peer dependencies that we need for Flower Shop include older versions of @angular/{core,http}, rxjs and zone.js.
Review the errors in the terminal output and add each missing dependency to package.json.
"peerDependencies": {
"@angular/core": ">= 2.0.0",
"@angular/http": ">= 2.0.0",
"rxjs": "^5.0.0",
"zone.js": "0.7.8"
},
08. Watch out for name changes
Our Flower Shop app makes use of an open-source custom component called ng2-modal. However, the author has since updated their naming convention to ngx-modal. We need to modify our dependency name and ng-module imports accordingly.
package.json:
"dependencies": {
[...]
"ngx-modal": "0.0.25",
[...]
},
app/app.module.ts:
import { ModalModule } from 'ngx-modal';
09. Check versions
Our custom modal component also needs updating to a more recent version, so be sure to update it with npm.
$ npm install ngx-modal@latest --save
10. Check your work
Now we’ve patched up our dependencies, it’s a good idea to clear node_modules and build it again from our package.json. If all goes well you should have a clean build! If you don’t, something may still be missing in your dependency versions. Take another look.
$ rm -rf node_modules/
$ npm cache clean
$ npm install
$ ng serve --open
Next page: Steps 11-20
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
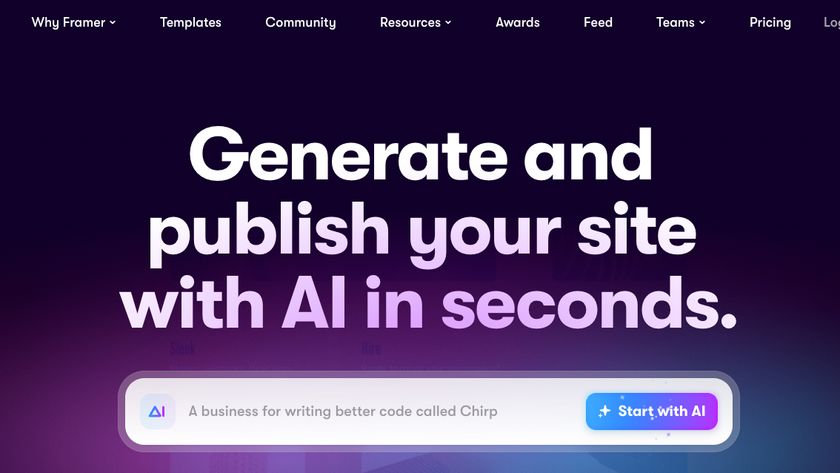
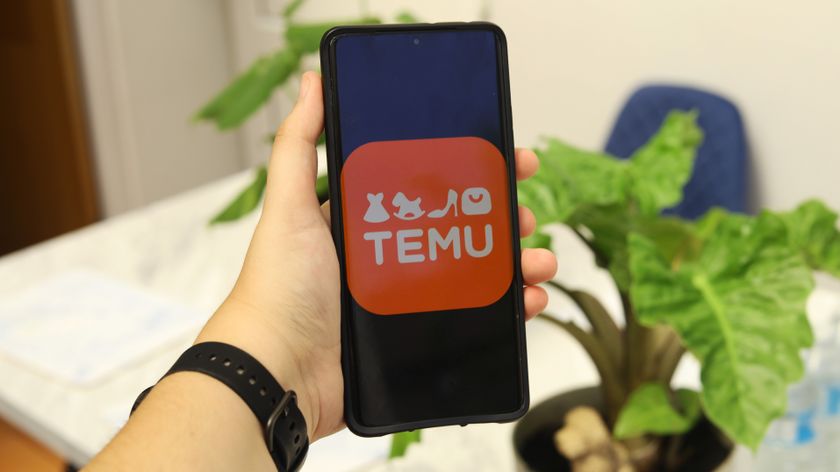
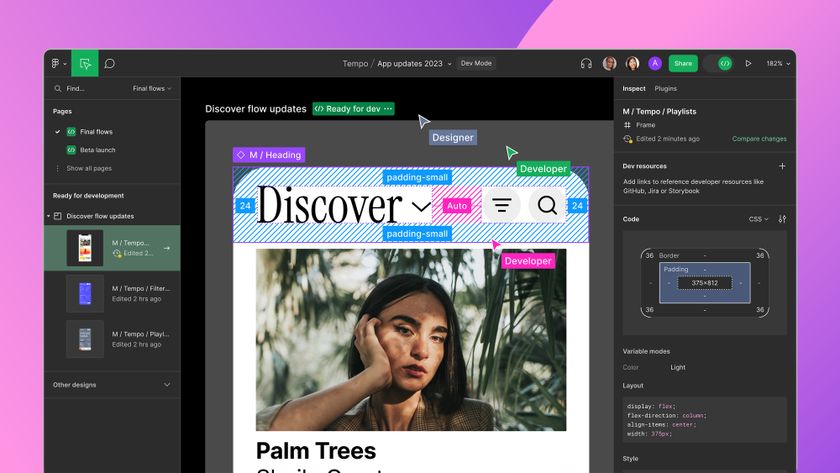
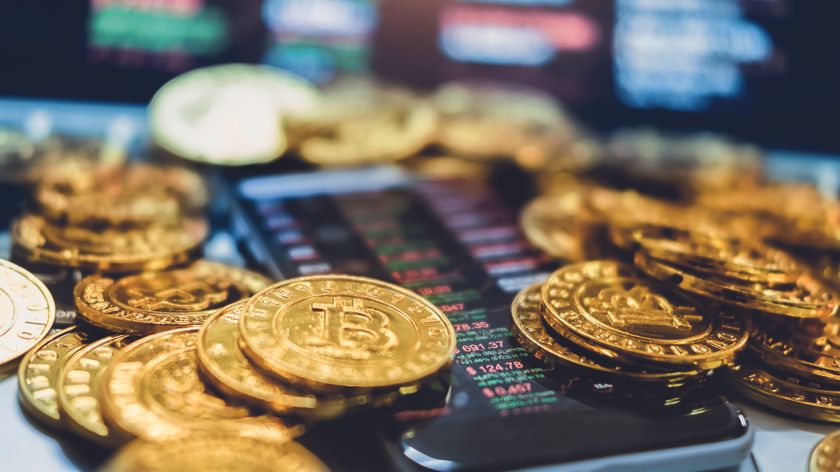
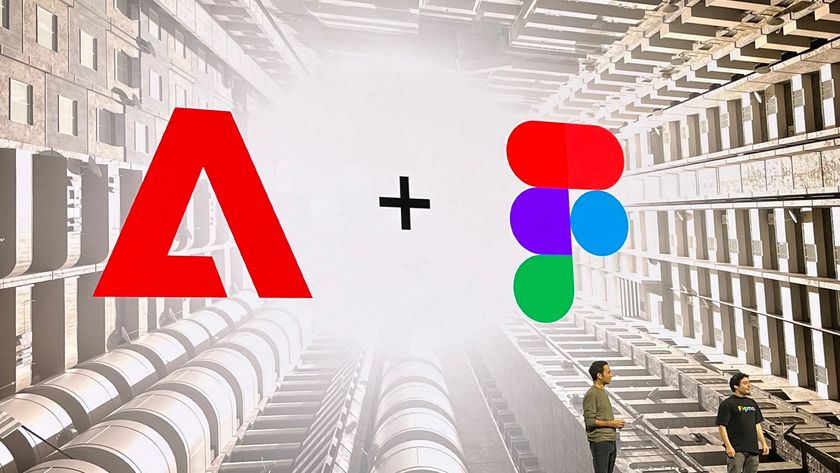
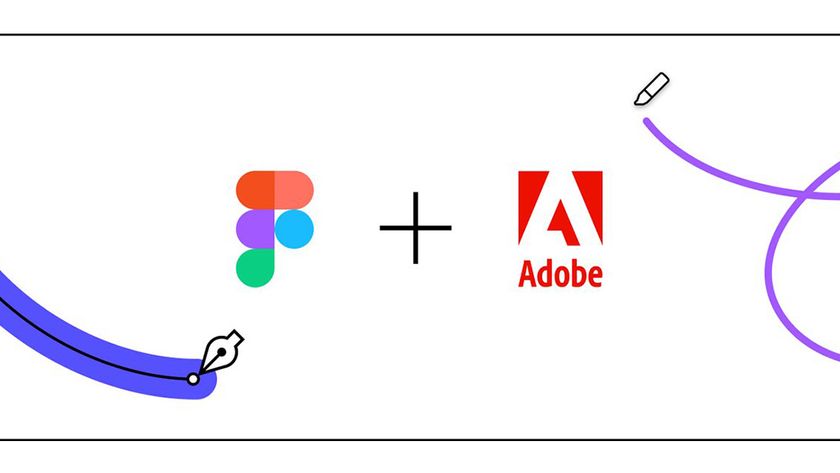
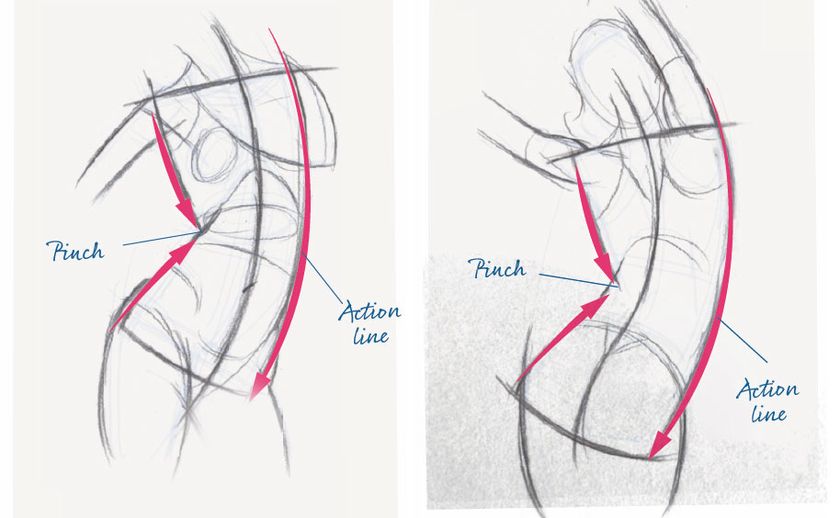
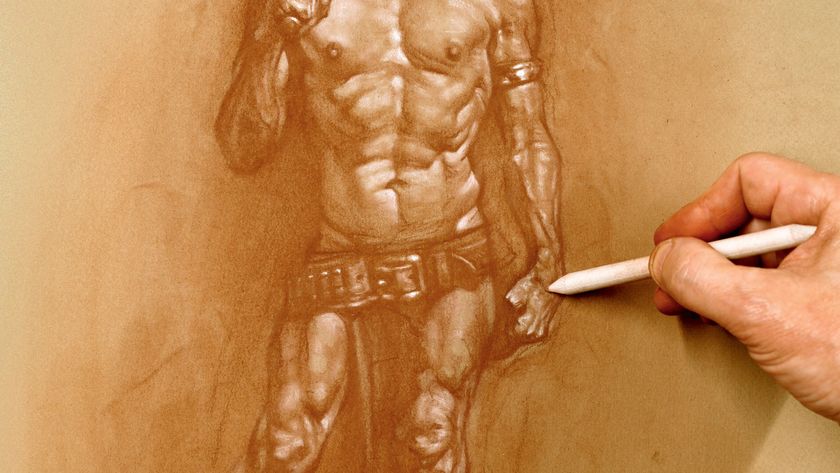
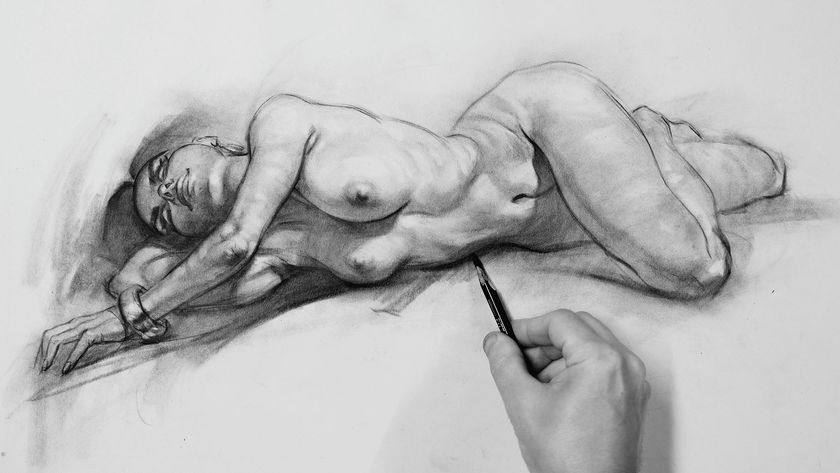
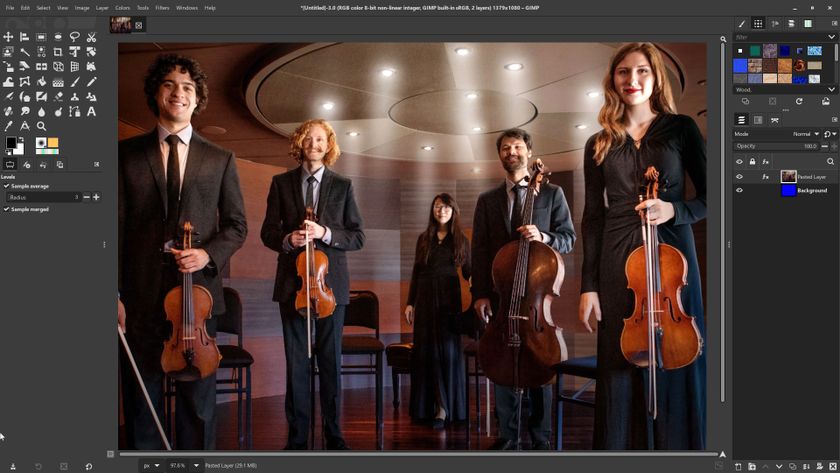
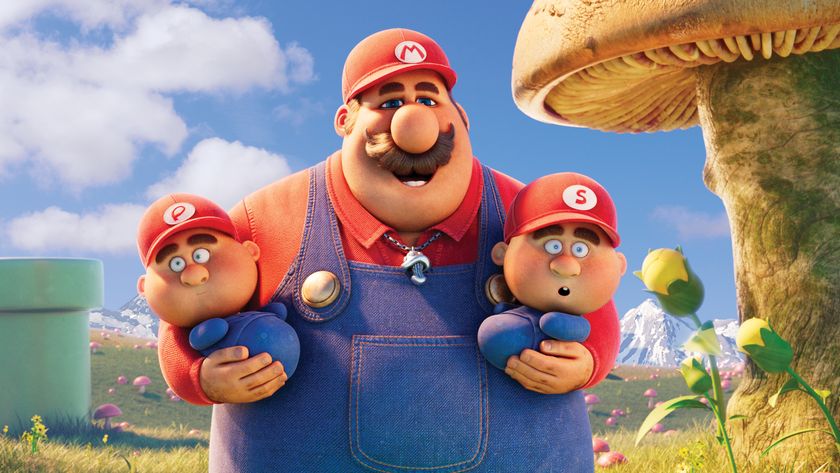
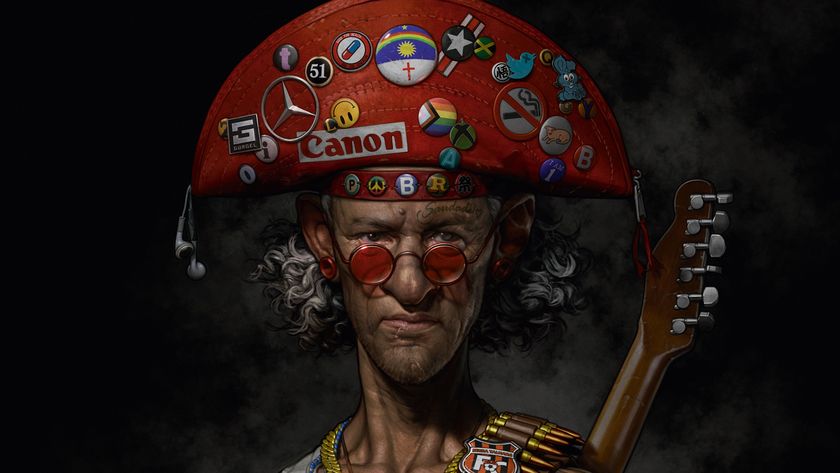