How to build a full-page website in Angular
Follow these simple steps to create a fully functioning website using Angular.
05. Generate the content service
In a production scenario, our content would probably come from a database, but for the sake of the article we are going to isolate our content to a service.
We can use the CLI to generate our content service, just like we did with our page component. We will create a shared directory, and then a services subdirectory, and then use ng g to generate our service.
mkdir src/app/shared
mkdir src/app/shared/services
ng g service shared/services/content
Unlike components, the CLI does not automatically add services to our Angular module, so we need to manually add it to the providers array of our metadata.
// app/app.module.ts
import { ContentService } from './shared/services/content.service';
@NgModule({
declarations: [...],
imports: [...],
providers: [ContentService],
bootstrap: [AppComponent]
})
06. Build out the content service
The CLI will generate a basic service stub that we can use to add in content for our pages.
ts
import { Injectable } from '@angular/core';
@Injectable()
export class ContentService {
constructor() { }
}
We are going to create a pages object with a key for each page we want to create and a value that represents the content of the page. This is going to be similar to the initial page object we created in our page component.
// app/shared/services/content.service.ts
import { Injectable } from '@angular/core';
@Injectable()
export class ContentService {
pages: Object = {
'home': {title: 'Home', subtitle: 'Welcome Home!', content: 'Some home content.', image: 'assets/bg00.jpg'},
'about': {title: 'About', subtitle: 'About Us', content: 'Some content about us.', image: 'assets/bg01.jpg'},
'contact': {title: 'Contact', subtitle: 'Contact Us', content: 'How to contact us.', image: 'assets/bg02.jpg'}
};
}
We can then pull our content from the ContentService by referencing the pages object with the appropriate key. In this case, we are hardcoding the key to home, but we will make it dynamic in just a moment.
// app/page/page.component.ts
import { Component, OnInit } from '@angular/core';
import { ContentService } from '../shared/services/content.service';
@Component({
selector: 'app-page',
templateUrl: './page.component.html',
styleUrls: ['./page.component.css']
})
export class PageComponent implements OnInit {
page: Object;
constructor(private contentService: ContentService) { }
ngOnInit() {
this.page = this.contentService.pages['home'];
}
}
It is considered best practice to keep initialisation logic out of the constructor as the code is hard to test, and sometimes data is not available immediately if it is coming from a parent component. The best place to put initialisation logic is in the ngOnInit method that gets fired after the component's bindings have initialised.
Get the Creative Bloq Newsletter
Daily design news, reviews, how-tos and more, as picked by the editors.
Page 3: Set up navigation routes between pages
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
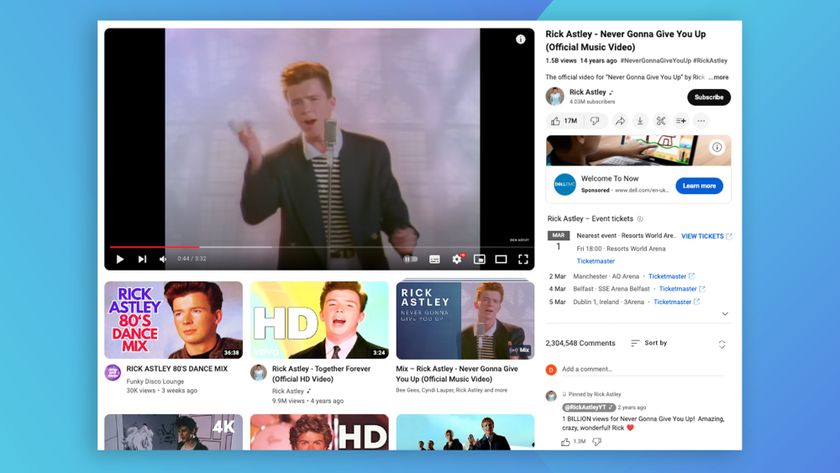
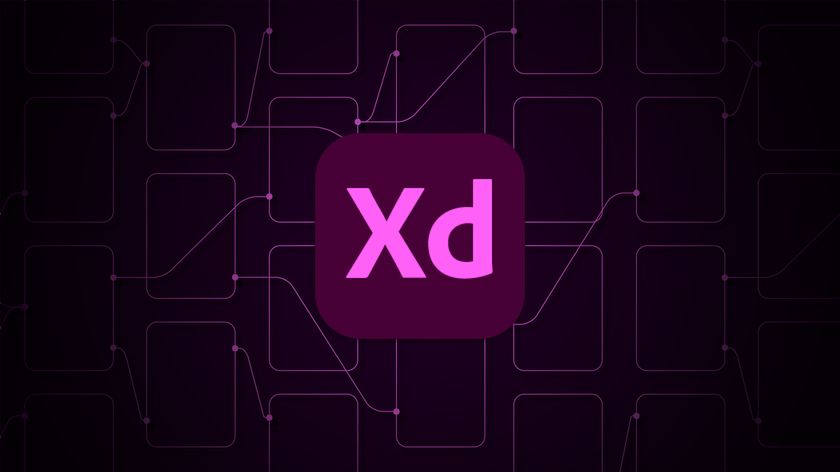
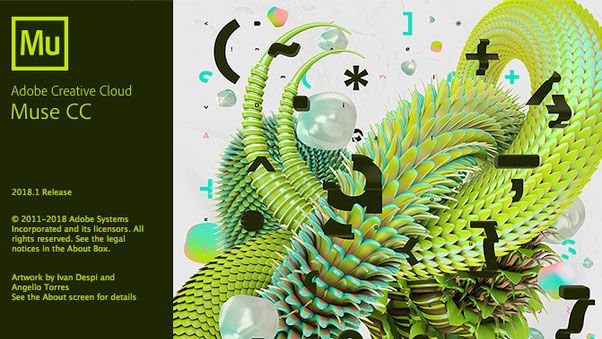
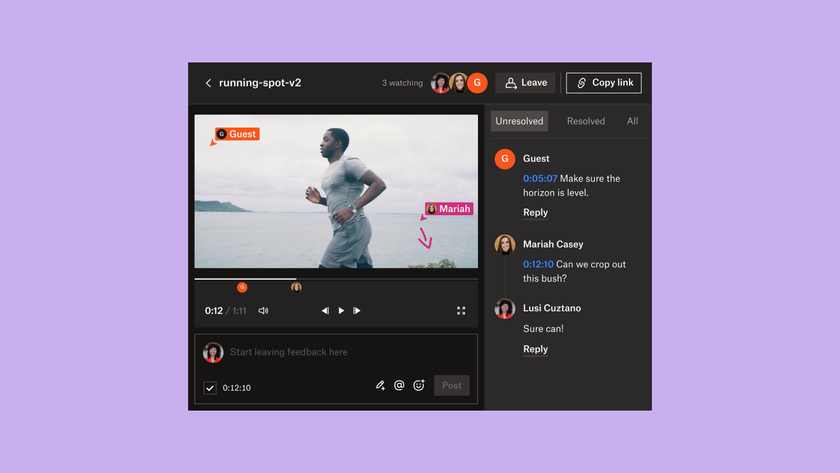
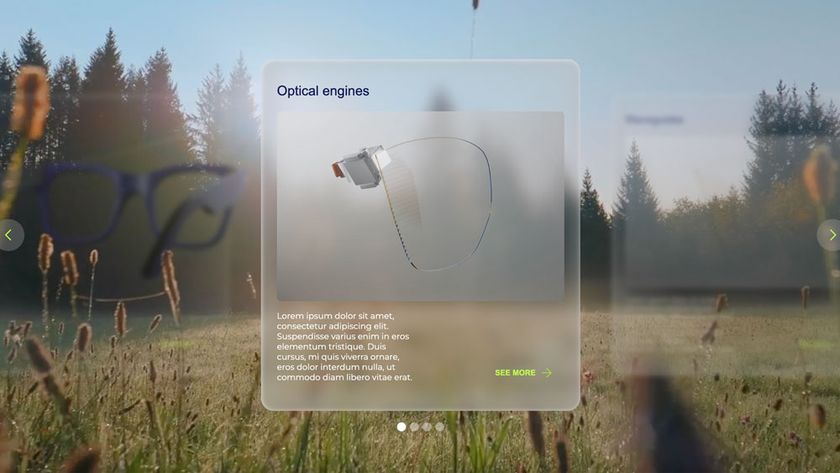
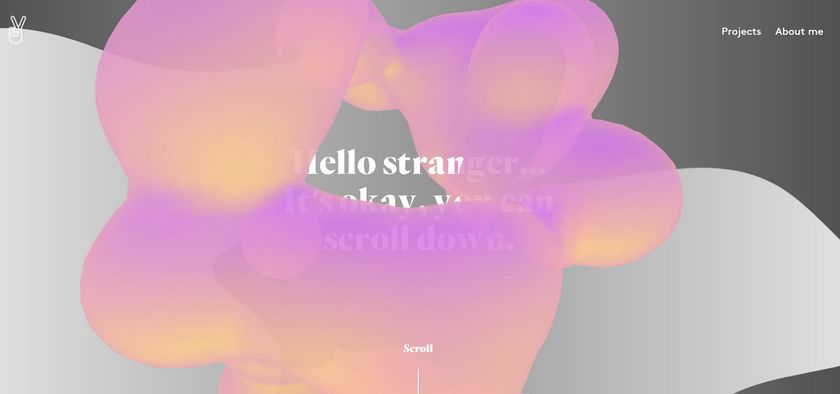
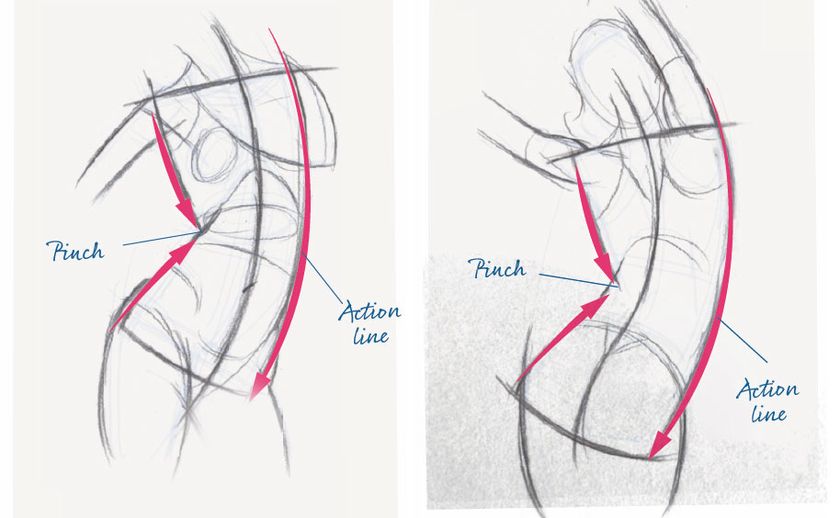
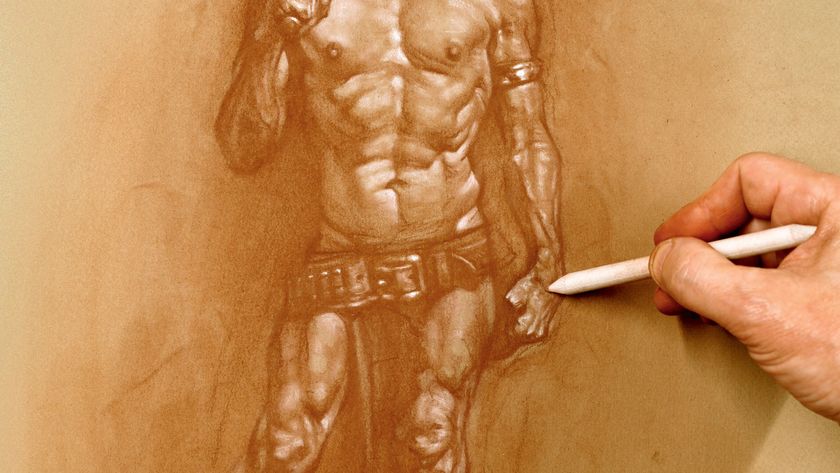
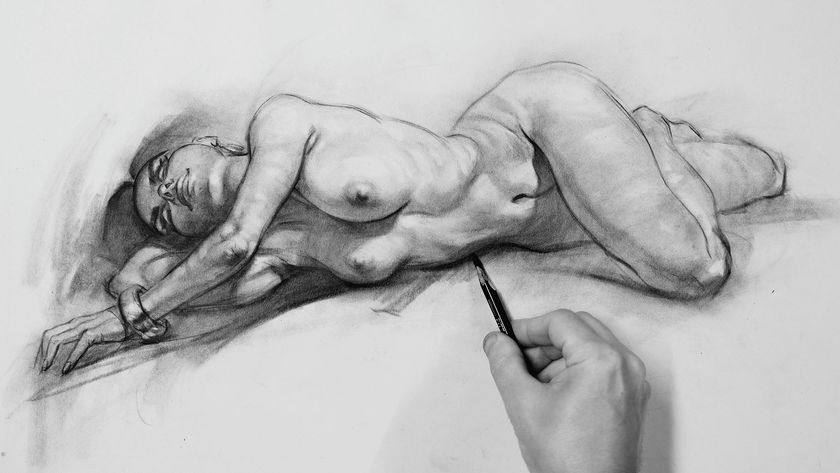
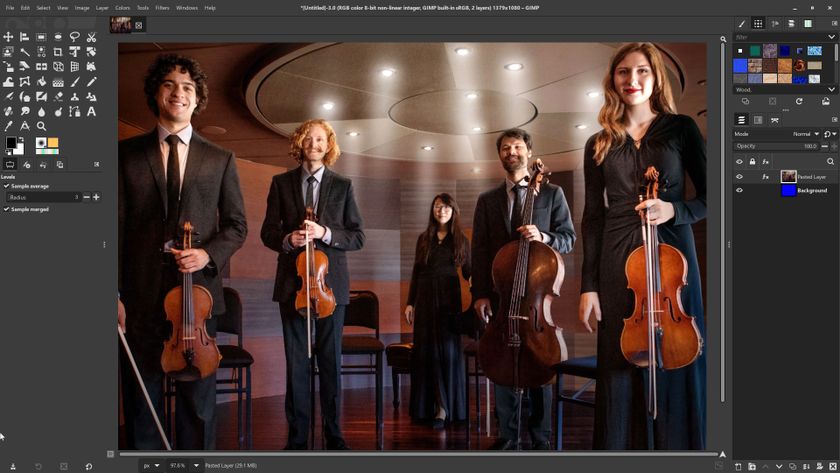
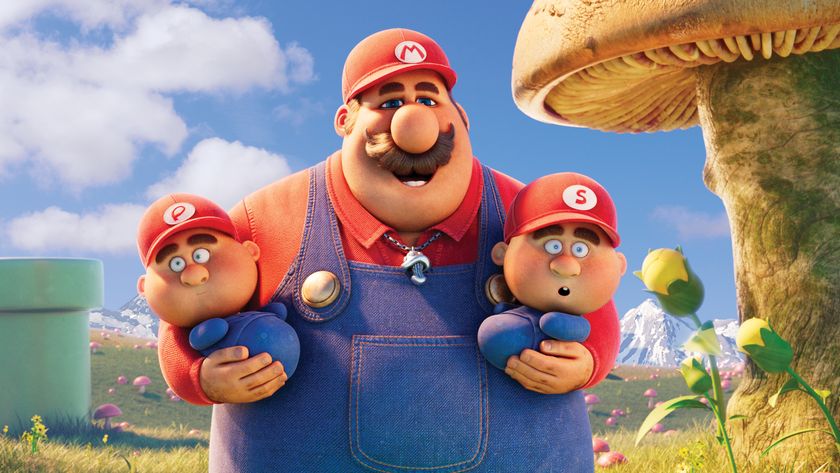
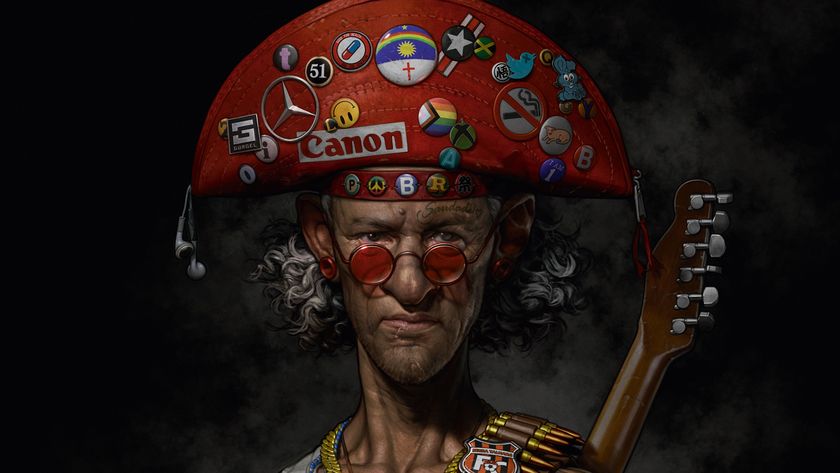