20 JavaScript tools to blow your mind
JavaScript has grown way beyond the browser. Discover the new features, tools and libraries transforming the way we use it.
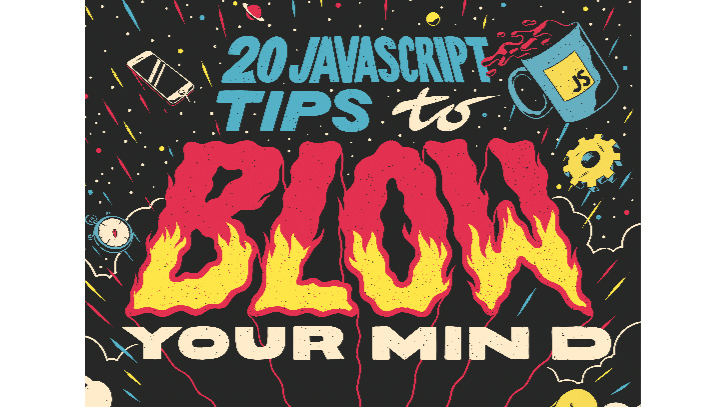
JavaScript started its life as a browser-based language used for adding interactivity to web pages, but it has evolved tremendously over the past few years. Once Node.js enabled JavaScript to be run on the server, it opened up a world of possibilities, and more language innovations were inevitable.
As the language grew, these evolutionary stepping stones led to the next major version of JavaScript: ECMAScript 2015 (previously known as ES6). Along with this new version came a new release cycle. After its major update in 2015, the JavaScript language will now start seeing smaller updates every year, allowing it to keep evolving through smaller, more frequent iterations.
Let’s take a look at some of my favourite JavaScript tips, tricks and tools. These will give you some web design inspiration and they're sure to blow your mind, especially if you haven’t kept up with how JavaScript has grown up over the years. These tools are split into different themes – navigate to the page you want using the drop-down menu above and keep your notes safe in cloud storage. Want a life without code? Try a website builder. However your site is built, make sure your web hosting is spot on.
01. Write tomorrow's JavaScript today with Babel
Not all browsers understand ES2015 code yet, so in order to use the latest features of the language today, we need a tool like Babel. This transforms ES2015 code into normal ES5 JavaScript code that all browsers are able to interpret. It is common for developers to include Babel in their deployment process through build systems such as gulp or webpack.
This approach allows devs to use the latest tech while ensuring their apps remain compatible with old browser versions, but only at the deployment stage.
02. Explore new ways of declaring variables
In ES2015, JavaScript introduced two new ways of declaring variables: let and const. let is used when a variable will be reassigned, whereas const keeps a variable from being reassigned. Note that using const does not freeze arrays and objects, and it doesn’t stop properties from being mutated. Instead, it just keeps the variable itself from being reassigned.
The main benefit that both let and const deliver over var is that when using var your variables get scoped to the top of the current function, therefore making the variable available to the whole function. In contrast, let and const are scoped to their closest block, allowing developers to declare variables within if, while, for and even switch blocks, without worrying about the variable scope leaking outside of that context.
Get the Creative Bloq Newsletter
Daily design news, reviews, how-tos and more, as picked by the editors.
03. Use arrow functions to keep 'this' intact
Another feature that’s been added to JavaScript recently is arrow functions. These have the ability to keep the this context intact, especially when using it within callbacks that might get called from somewhere else (i.e. adding an event listener with jQuery, and so on). Essentially, arrow functions replace the need to add .bind(this) at the end of a function declaration.
There are two main ways of writing arrow functions: one-liners and multiple-liners. One-liners have only one expression and return the value of that given expression, without the need for curly braces. Multiple-liners, on the other hand, have curly braces and the return keyword must be used explicitly.
let doubleShort = (num) => num * 2;
let doubleLong = (num) => {
let doubleNum = num * 2;
return doubleNum;
}
04. Use promises to avoid a callback can of worms
JavaScript does a lot of its operations asynchronously, so passing callback functions while waiting for other things to happen is a pretty standard pattern. The problem begins, though, when you’re executing an async action that will trigger another async action, and so forth. You’ll likely be passing many nested callback functions, which will make the code cluttered and harder to read.
Promises solve this problem by helping you execute code in the right order, in a concise manner, while keeping operations asynchronous. The API is pretty neat: you tell the code to do something, then something else, then something else – and you’re even able to catch errors along the way.
Next page: Functional programming tips
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
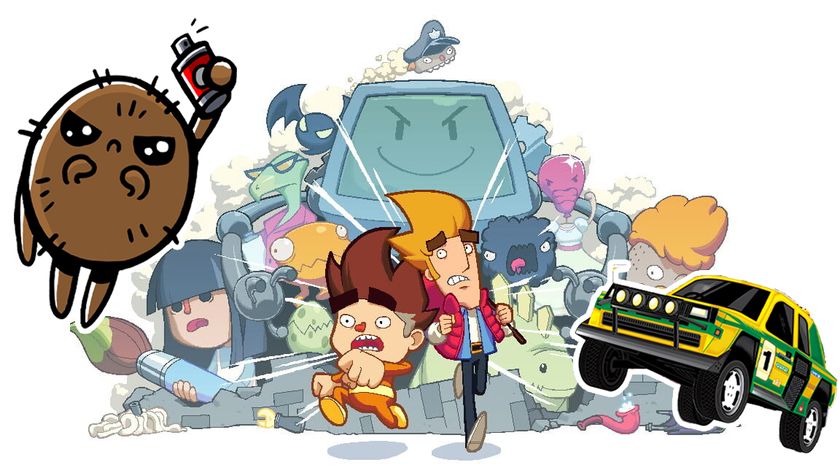
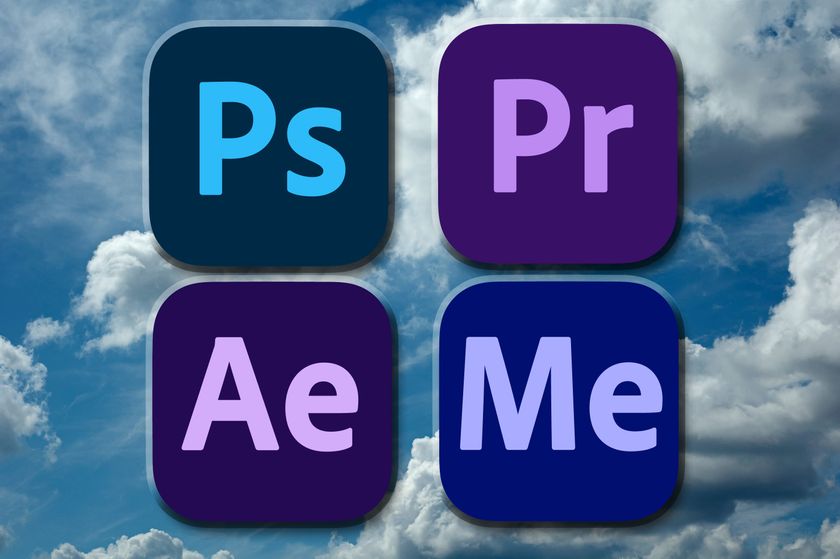
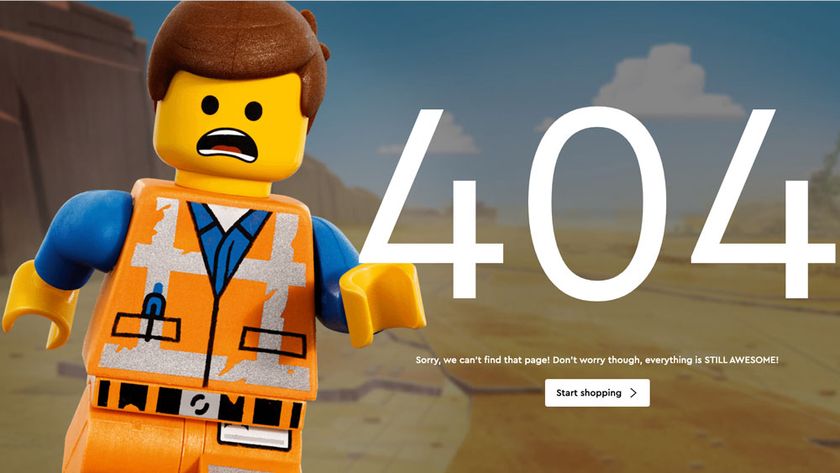
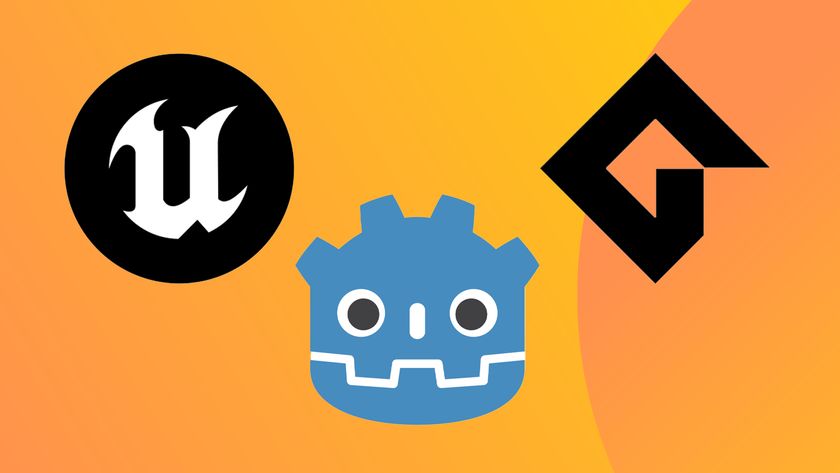
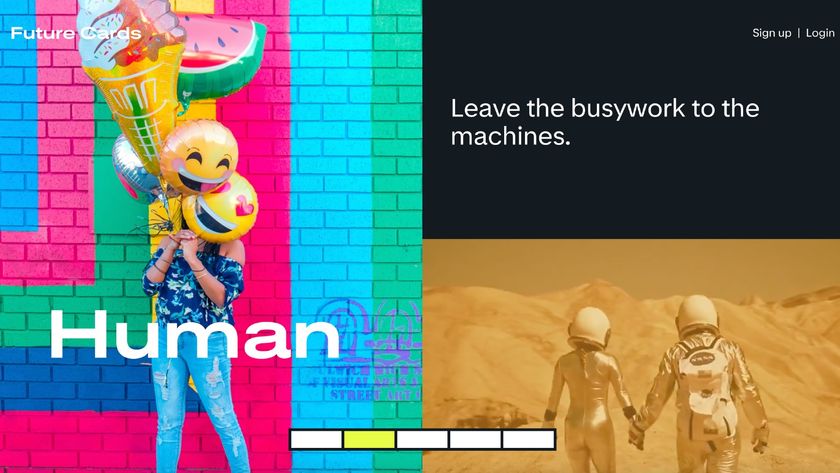
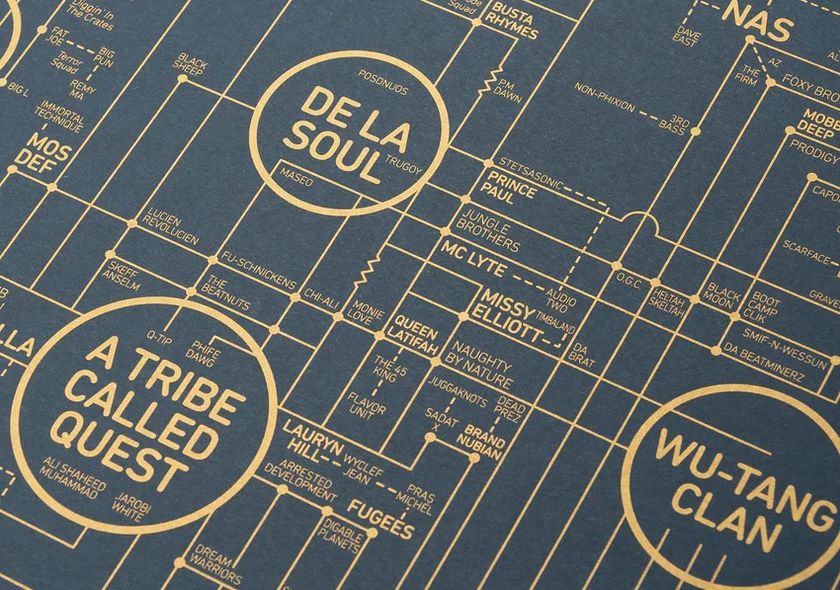
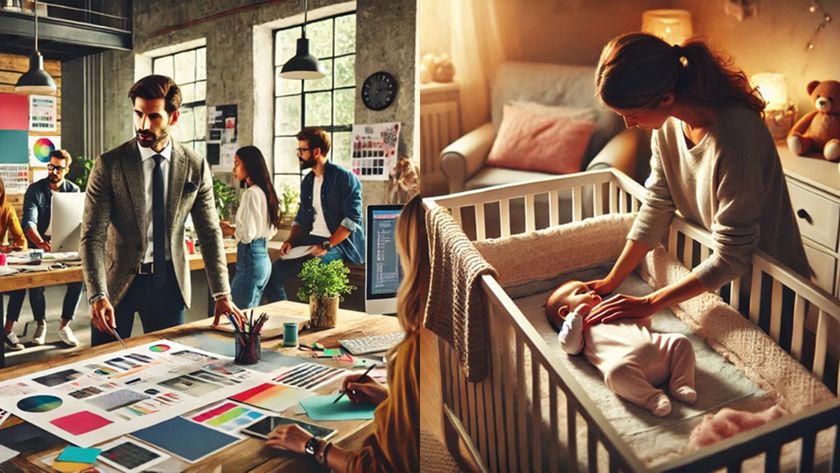
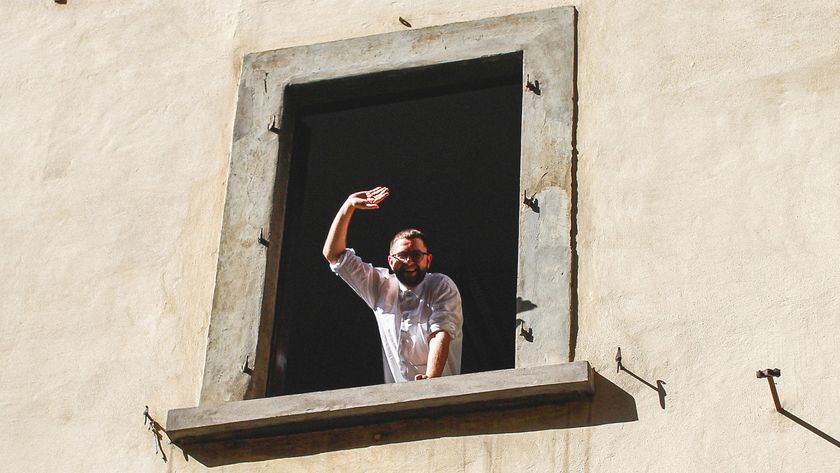
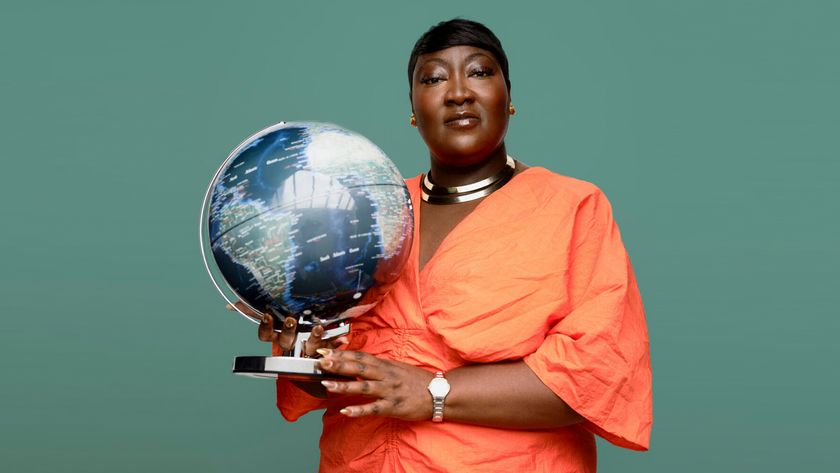
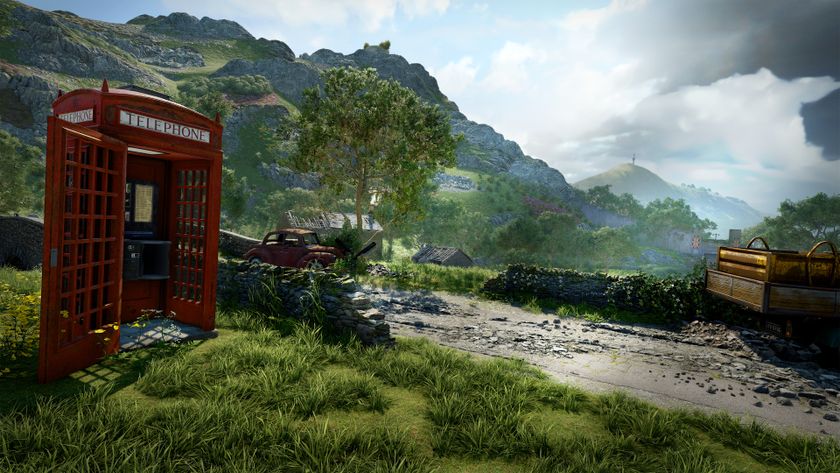
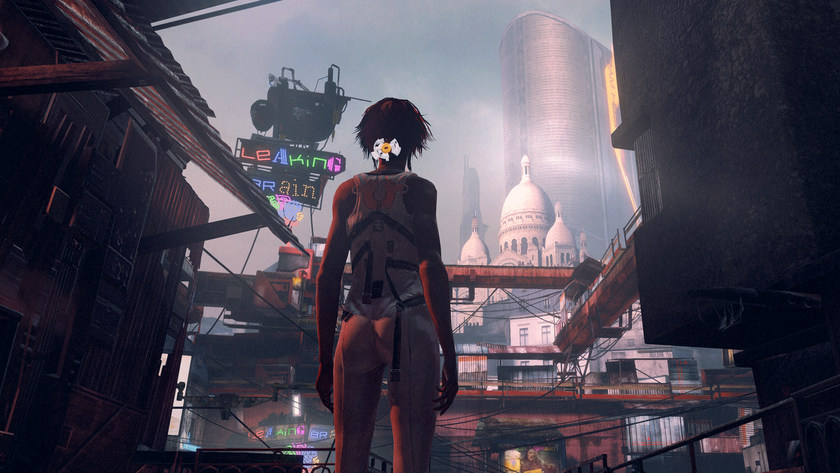
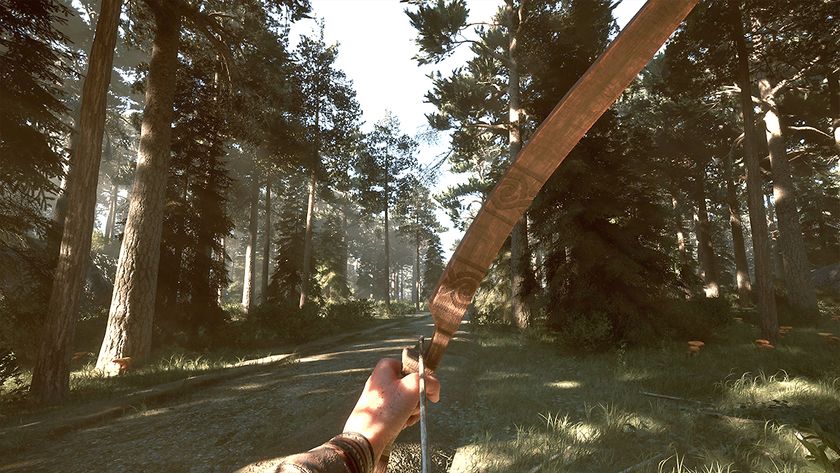