15 essential JavaScript tools you should be using
The hottest frameworks and tools you need to try out.
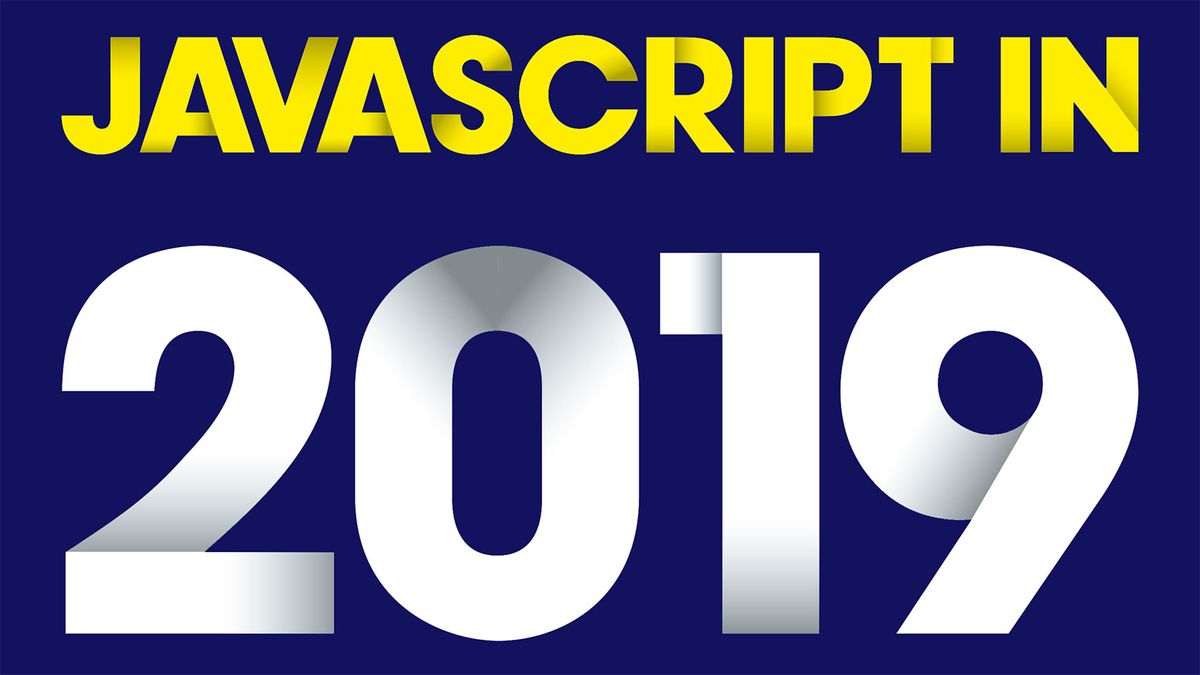
JavaScript is a vital tool for any web developer. To help you get the best from this powerful programming language, you're going to want to enlist the help of some dedicated tools (and get your web hosting right). The good news is, you're not short of options here: there are plenty of JavaScript tools around to choose from. The difficult part is picking the ones that are right for you and your workflow.
In this article, we've rounded up what we think are the best JavaScript tools around right now. If you're not a developer, or just want to create a site quickly and without the need for code, consider using a website builder.
01. Web Components
The concept of a component is used heavily in JavaScript frameworks. They are used to break apart a project into several reusable chunks that can be moved about independently of one another. But what happens when it’s time to change the framework? Previously written components will no longer work. Either there is added workload to convert the components, or the project stays tied to that framework.
Web components are a solution to that issue. They are written using native web technologies, are self-contained and expose values and behaviours through attributes like any other HTML element.
They are made up of three separate specifications: HTML templates, shadow DOM and custom elements.
HTML templates
These define the visual appearance of a component. They contain the structure of content, which can then be copied and reused for each component on the page.
Shadow DOM
The concept of keeping the internal markup of the component away from the rest of the document. This keeps styles and other logic from breaking out and affecting other components.
Get the Creative Bloq Newsletter
Daily design news, reviews, how-tos and more, as picked by the editors.
Custom elements
These are the glue that holds everything together. They are responsible for the lifecycle of the component, which can include extra logic for when a component is added or removed from the page.
Web components can be used alongside their framework counterparts. As they are supposed to act like native elements, most frameworks will work with them without issue. Some frameworks, like Angular, may need to be told of their existence ahead of time to function properly.
As they can be readily composed together, components can be imported from many sources. Websites like webcomponents.org provide several ready-made elements that can be dropped in and used straight away.
While the concept of a web component has been around since 2011, the specifications have constantly changed. In 2018 things settled down, with Firefox implementing the latest versions of shadow DOM and custom elements specifications in October. With Edge due to ship its implementations in 2019, all major browsers will natively support them.
02. WebAssembly
One complaint about using the browser to host applications is that JavaScript runs too slowly when it comes to processor-intensive tasks, such as game engines or video editing.
WebAssembly – or ‘WASM’ – is a compile target for code written in other languages more suited to heavy lifting. This enables existing programs written in languages like C++, Rust or Go to make it to the web. By compiling these down into a binary format, it enables them to be split into chunks and downloaded efficiently.
These binary files are then compiled in memory and instantiated using the new WebAssembly object. There is a push to make these importable like any other JavaScript modules, but work in this area is still ongoing.
While WASM is a new language to work with in the browser, it does not make it a competitor to JavaScript. Both languages have their benefits, with JavaScript still lending itself better to day-to-day operations in the browser. They can work together and have autonomy over separate parts of an application.
WASM has cross-browser support, now including mobile. This opens the door to many opportunities that were previously limited to native applications. Over the next year we may see more games and application developers embrace this technology.
03. Vue
Vue has been steadily on the increase in the past year. Recent updates have had more of a focus on developer experience and quality improvements. Vue 2.5 was released back in 2017 and included updates to error handling, server rendering and TypeScript definitions. Single functional components – one of Vue’s more popular features – were updated to support scoped CSS and also improved template compilation.
Since then, updates to the core Vue experience have been mostly focused on bug fixes and performance improvements. The focus has now shifted to complementary development products.
Version 3 of Vue’s CLI tool moves to a more configurable approach. Features such as routing, linting and data management are selected during initialisation. These features can be added and removed as needed without picking apart the build process.
Along with the CLI, there is a web interface available to visualise the different parts of an application. This acts as a dashboard to help analyse load times, sizes and pain points within the build as it runs.
Looking forward, 2019 will see the release of Vue 3.0. While breaking changes will be kept to a minimum, this version will focus on making use of ES2015 class-based components. It will also support fragments and portals, much like the features in React.
- Learn more: Speed up performance with Vue.js
04. TypeScript
Bring type safety to JavaScript with TypeScript. This tool enables you to define expected types for variables and have build tools and IDEs warn you of any issues. Version 3 delivers better support for newer concepts, such as rest and spread operators.
- Learn more: How to get started with TypeScript
05. React
React 16.6 brought the ‘suspense’ feature, which made dynamic content easier to work with. Combined with React.lazy(), it creates a point at which to split code into smaller chunks. Suspense can render a fallback component, such as a loading spinner, while the component loads. In 2019, suspense will grow into a more flexible tool. By triggering data fetching as part of the flow, users will get a seamless, native-like experience.
One of the easiest ways to get up and running with React is by using the ‘Create React App’ tool to stamp out a project. A recent update upgraded its dependencies and improved its features. Create React App 2.0 uses Babel 7 and Webpack 4 to build projects, which makes it faster to build and opens the door to newer features like the shorthand fragment syntax.
Projects made with the previous version can be upgraded by updating the ‘react-scripts’ dependency. Updating ejected applications is a more manual process, but they can be upgraded piece by piece.
React shows no sign of slowing down this year. Hooks enable functional components to state and lifecycle behaviours. Concurrent mode improves the performance of slow-rendering components. Both features are slated for a release in the first half of 2019.
- Learn more: Develop reusable React components
06. Angular
Angular is a framework involved in all parts of an application, including data handling and interface updates. While it can inflate bundle sizes, all parts of the application will work together seamlessly.
Version 7 of Angular adds improvements to every part of the framework. While there are few changes that developers will get to play around with, there are lots of changes to benefit speed and reliability behind the scenes. It is now possible to add performance budgets for bundle sizes. This will make sure that a build never gets excessively large without being made aware.
As part of an overall update to Material Design, the respective Angular CDK components have also been updated. For example, the scrolling module enables support for virtual scrolling, which helps to keep long lists scrolling smoothly.
Upgrading to version 7 will, for most, involve running one line using the CLI.
ng update @angular/cli @angular/core
Future updates will focus on the new Ivy rendering engine. This will improve the ability to remove unused code from the bundle, resulting in dramatically reduced file sizes. Due to the structure of Angular applications, the rendering engine can be replaced without requiring any changes to the internal logic.
07. Polymer
In its first iteration, the Polymer library was the gateway to a future of custom elements. By embracing the then-upcoming web component specifications, developers were able to piece together websites from ready-made building blocks.
Over time, these specifications have evolved, and one of the building blocks – HTML imports – was no longer part of the plan. The recent release of Polymer 3.0 addresses this issue and focuses more on using more approachable ES modules to achieve a similar goal.
The original aim of the Polymer library was to become lighter as the support for those specifications grew, but it ended up growing instead. To refocus on that aim, 3.0 is the last version of the library to be released. Moving forward, it is recommended components are made with LitElement and lit-html.
class MyElement extends LitElement {
render(){
return html`
<div>This is a LitElement
</div>
`;
}
}
LitElement is a lightweight wrapper around web components to make them easier to work with. The html tagged template literal is lit-html, which is a templating library with DOM diffing to keep page updates as small as possible.
Both LitElement and lit-html are in a pre-release state at the moment, but the Polymer team aims to get them released at some point early this year.
08. Prettier
This JavaScript tool is dedicated to ensuring code formatting stays consistent across a project. Have Prettier comb over files and update the formatting automatically. Some editors also support formatting on every save.
If you're working on a team project, make sure your process is totally cohesive with the perfect cloud storage option.
09. Service Workers
Service workers enable an application to run a background process that manages some lower-level features. This can include push notifications, syncing data in the background and even providing an experience for when the device is offline. The level of control is up to the developer.
Service workers have been around for some time on Android, but they have also recently made the jump over to iOS. While the focus is on mobile devices, they also work on desktop. Now that the majority of visitors will have access to the functionality, there is no better time to start improving the experience for users.
- Learn more: Make your app work offline with service workers
10. OffscreenCanvas
There are times when a canvas element is the best tool for the job. For example, online games can use them to generate sprites, or videos can have processing effects applied to them.
The problem is that any interactions with the element will always work on the main thread, which slows things down for the user. For repeated animations or heavy processing, this can became a problem.
OffscreenCanvas decouples the canvas logic from the element. Without the link to the DOM, it can be used in a worker to free up the main thread. Currently only Chrome supports this feature, with Firefox including it behind a flag.
11. Electron
Use HTML, CSS and JavaScript to build native applications for Windows, MacOS and Linux. Electron powers many popular applications, such as Visual Studio Code, Slack and Skype for desktop.
12. Svelte
While Svelte is a relatively unknown framework, it has been steadily gaining traction since its release a couple of years ago. The recent State of JavaScript survey ranked it more popular last year than the historic titans of Backbone and jQuery.
The aim of Svelte is to keep the file size down by not shipping a framework at all. As part of the build process, it analyses the components and optimises them before compiling to vanilla JavaScript. The result is an application with the smallest possible size.
With version 2, there are improvements to syntax to make components as readable and predictable as possible. Updates to lifecycle hooks and computed values make it easier for the compiler to know where to make optimisations.
Features are deliberately light to keep each application fast. Many updates since version 2 have focused on avoiding situations that result in poor performance in the compiled output.
Discussions are being held about a rewrite of component logic in Svelte to bring it in line with other frameworks. As a compiler, it is able to make its own modifications to help function better, while remaining a clean environment for developers.
13. Resize Observer
A truly component-based approach to web development is closer than ever. While CSS and JavaScript can be bundled in one package, it’s difficult to then reuse that component reliably across pages.
When adding elements to a resize observer, it gets notified when the bounds of the element change. The visuals can then be updated based on the space available. As an example, the same card component can look different in either a sidebar or the main body.
This observer is currently only available in Chrome, while it is in development in Firefox. Other browsers can fall back to monitoring browser resize events, but this will hit performance.
14. Cordova
Build multiple different mobile and desktop applications using web technologies and one codebase with Cordova. PhoneGap – Adobe’s distribution of Cordova – provides additional tools, such as iOS development on Windows.
15. Storybook
Storybook enables you to create a gallery of UI elements in a customisable environment separate from any application. It works with popular frameworks such as React and Vue, along with support for HTML snippets.
This article was originally published in creative web design magazine Web Designer. Buy issue 283 or subscribe here.
Read more:
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
Matt Crouch is a front end developer who uses his knowledge of React, GraphQL and Styled Components to build online platforms for award-winning startups across the UK. He has written a range of articles and tutorials for net and Web Designer magazines covering the latest and greatest web development tools and technologies.