Colour theming with Sass and Compass
Ben Frain demonstrates some nifty features of Sass and Compass that make colour manipulation quick and simple
One Sass feature that typically piques the interest of designers is the ability to manipulate colour. Ordinarily, with 'vanilla' CSS, unless you're familiar with hex, RGBA or HSLA values, it can be difficult to make rapid adjustments to colours in the code.
For example, without opening a colour picker, can you define the hex value of #ff9900 but 10 per cent lighter? No? Too slow! How about that value tinted 30 per cent? No? This is exactly the kind of manipulation you can do in the code with Sass and Compass in an instant.
For example, here's the first conversion addressed with Sass:
.no1-challenge { background-color: lighten(#ff9900, 10%);}
And that results in this in the compiled CSS:
.no1-challenge { background-color: #ffad33;}
And here's the second challenge dealt with by Sass and Compass:
.no2-challenge { background-color: tint(#ff9900, 30%);}
Which results in this in the compiled CSS:
.no2-challenge { background-color: #ffb74c;}
Some Sass fundamentals
Before we look at the powerful colour manipulation functions of Sass and Compass be aware that, for this piece, we'll skip over most of the Sass and Compass basics. That way we can cover the colour related features in sufficient detail. Therefore, if any of the Sass syntax being used here seems alien, perhaps treat yourself to a book on the subject. I know a good one! That said, before we get into the thick of things, we'll cover a few essentials just to refresh your memory. First of all, variables.
Get the Creative Bloq Newsletter
Daily design news, reviews, how-tos and more, as picked by the editors.
Variables
It's not necessary to save and recall colour values by their hex, HSLA or RGBA value when using Sass. For simplicity, we can store the value in a variable. Variables in Sass work like this:
$variable-name: variable-content;
The variable syntax is that the dollar sign indicates the start of a variable, the colon indicates the end of a variable name and the part after, before the semi-colon, is the variable's value.
Storing colour values in variables
With that covered, we will store our prior colour value as a variable:
$bf-orange: #ff9900;
Excellent. We have now abstracted our colour into an easier to remember variable name. You may prefer to name colours as variables sequentially:
$color1: #d90000;$color2: #59B200;$color3: #00B2B3;
Or with names that are relevant to your design:
$primary-branding: #d90000;$secondary-branding: #59B200;$tertiary-branding: #00B2B3;
Whatever makes most sense to you.
Now let's press on and see what Sass and Compass can do for us.
Sass and Compass colour functions
Sass has a number of colour manipulation functions and Compass adds yet more. The full list of Sass colour functions can be found at the Sass documentation pages. The Compass colour helpers are documented on the Compass website.
Let's look at some colour manipulations from both Sass and Compass.
RGBA Function
First off, let's try making one of our hex colours (stored as the variable $bf-orange) slightly transparent:
.rgba { background-color: rgba($bf-orange, .5);}
In this example, we're using the Sass rgba function. After declaring the function within the brackets, we pass the 'arguments' for the function. The first argument is the colour we want to manipulate (it can be passed as a variable, hex, RGBA or HSLA value) and then the quantity of the manipulation. With the rgba function, it can be any integer value between 0 and 1. Here is the result as compiled CSS:
.rgba { background-color: rgba(255, 153, 0, 0.5);}
Hopefully you can see what's happened there? Sass has converted the colour into an RGB value complete with an Alpha channel that matches the value passed as an argument.
Mix function
How about mixing two colours together? Yep, Sass has you covered. Here's an example:
.mix { background-color: mix($color1,$color2,20%);}
That results in the following CSS:
.mix { background-color: #8c6a00;}
Here's how the function works. After declaring the mix function, we pass three arguments. The first two arguments are the colour values you'd like to mix (remember you can pass the colours as variables or hex, RGBA or HSLA values). The third argument is the amount of the first colour you wish to mix into the second. In our prior example, we're mixing 20 per cent of $color1 into $color2.
Saturate and desaturate functions
As the saturate and desaturate functions are inextricably linked, let's cover them in one go. Hopefully, you can guess at how the syntax will work by now:
.saturate { background-color: saturate($bf-orange, 20%);}
Like the prior Sass functions we've looked at that work with a single colour, after declaring the function name, within the brackets that follow the arguments passed are the colour to manipulated and then the amount of manipulation.
The desaturate function works exactly the same way:
.desaturate { background-color: desaturate($bf-orange, 20%);}
Nesting colour functions
I sense you're feeling a little at home with this now, so let's shake things up a little. Let's nest a couple of colour functions within one another. Here's one way Sass lets you do that:
.saturate-and-mix { background-color: saturate(mix($color1,$color2,20%),20%);}
You might feel that looks a little bonkers (that's a technical term), so let's break that down. All that we've done is nest one colour function within another. So, we've started with the first colour function (in this case saturate) but instead of passing the first colour as a hex, RGBA, HSLA value or variable, we passed another colour function: the mix based example we have already used. That compiles to the following hex value in the CSS:
.saturate-and-mix { background-color: #728e00;}
Now, if nesting colour functions in that manner looks too confusing, you could always store the first value (in this case the result of the mix function) as a variable. Here's how:
$desaturate-as-variable: mix($color1,$color2,20%);.desaturate-and-mix { background-color: desaturate($desaturate-as-variable, 20%);}
Automatic taste for colour dunces
I'll level with you; I'm terrible with colour. I have trouble knowing which colours go with others. Mercifully, if you have the same problem, thanks to mathematics and Sass we can enjoy some quick wins. This could make you feel a little more heroic.
Complement function
Given one colour, the Sass complement function can auto-magically (that's another technical term) return you a complementing colour. Let's give it a whirl.
.complement { background-color: complement($bf-orange);}
The complement function only requires a single argument: the colour you want to complement. Here is the CSS that compiles to:
.complement { background-color: #0066ff;}
The visual result is a blue that, as you might hope, complements the orange perfectly (download the example files to see for yourself).
What witchery is Sass employing to make this happen? It turns out that the complement is a shortcut for adjusting the hue of a colour in HSL colour space by 180 degree.
Adjust-hue function
The same effect as complement can be achieved by using the Sass adjust-hue function:
.adjust-hue { background-color: adjust-hue($bf-orange, 180);}
That compiles to same hex colour value:
.adjust-hue { background-color: #0066ff;}
The adjust-hue can be used to effectively rotate the HSL colour wheel negatively or positively. Here's an example using a negative rotation:
.adjust-hue-negative { background-color: adjust-hue($bf-orange, -30);}
In that example we're manipulating our colour minus 30 degrees on the HSL colour wheel. That results in a red colour with the following hex value:
.adjust-hue-negative { background-color: #ff1a00;}
Lighten and darken
Another two functions that manipulate colour in HSL colour space are lighten and darken. The syntax should now be familiar:
.lighten { background-color: lighten($bf-orange, 20%);}
That results in this CSS:
.lighten { background-color: #ffc266;}
Darken works in exactly the same way:
.darken { background-color: darken($bf-orange, 20%);}
And results in this CSS:
.darken { background-color: #995c00;}
As you can imagine, these two functions work by manipulating the lightness of a colour in HSL colour space. Again, you can see the results in the download files.
The Compass tint and shade functions
The authoring framework Compass adds two particularly useful functions: tint and shade. You may wonder how these two differ from lighten and darken. While lighten and darken alter the lightness or darkness of the colour, tint and shade alter the value of a colour by adding white (tint) or black (shade) to the colour being manipulated. This turns out to be very useful when building out code from graphical composites. It's common for highlight and lowlight lines to be added to UI elements in Photoshop by using a white or black line over the edge of an element. Transparency is applied to the line to allow the background colour to be partially visible. Shade and tint provide a code-based equivalent of this. Here's an example:
.tint-and-shade { border-top: 15px solid tint($bf-orange, 20%);background-color: $bf-orange;border-bottom: 15px solid shade($bf-orange, 20%); }
With the tint function, the percentage passed in the arguments is the amount of white the colour should be mixed with. With the shade function, the percentage is the amount of black the colour should be mixed with.
The prior example results in the following CSS:
.tint-and-shade { border-top: 15px solid #ffad33; background-color: #ff9900; border-bottom: 15px solid #cc7a00;}
Summary
There are other simple colour manipulation functions like grayscale and slightly more complex functions, including adjust-color and scale-color, which are available to you when working with Sass and Compass. As mentioned previously, remember that any of these manipulations can also be nested within one another for some pretty complex manipulations. Try nesting colour manipulations in a colour picker!
By being aware of what Sass and Compass can achieve in the code, it allows for far easier colour theming. Even when the colours needed for elements are unknown, these colour functions allow rapid prototyping by running with some trusted colour manipulations. Even with only a single colour defined, it's easy to create colours that will work alongside without ever leaving the comfort of your coding environment.
Thank you for reading 5 articles this month* Join now for unlimited access
Enjoy your first month for just £1 / $1 / €1
*Read 5 free articles per month without a subscription
Join now for unlimited access
Try first month for just £1 / $1 / €1
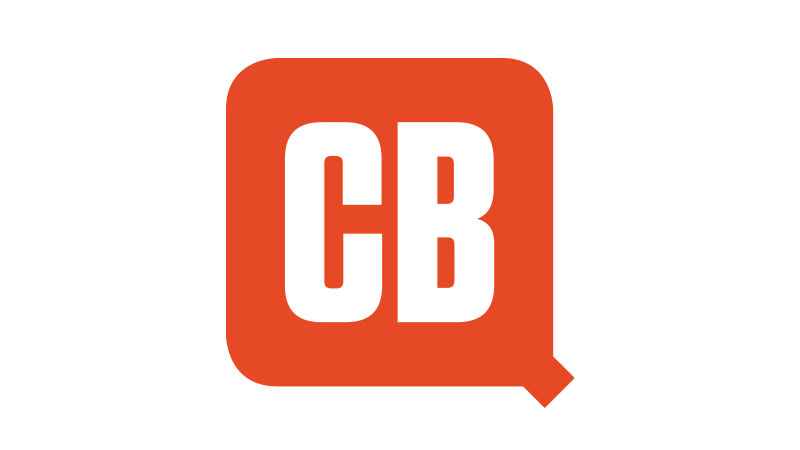
The Creative Bloq team is made up of a group of design fans, and has changed and evolved since Creative Bloq began back in 2012. The current website team consists of eight full-time members of staff: Editor Georgia Coggan, Deputy Editor Rosie Hilder, Ecommerce Editor Beren Neale, Senior News Editor Daniel Piper, Editor, Digital Art and 3D Ian Dean, Tech Reviews Editor Erlingur Einarsson, Ecommerce Writer Beth Nicholls and Staff Writer Natalie Fear, as well as a roster of freelancers from around the world. The ImagineFX magazine team also pitch in, ensuring that content from leading digital art publication ImagineFX is represented on Creative Bloq.